Customizing widget behavior with PyQt event handling
Write a Python program that overrides a specific event handler to customize widget behavior using PyQt.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QWidget: The QWidget class is the base class of all user interface objects.
Qt module: PyQt5 is a set of Python bindings for the Qt application framework. It allows us to use Qt, a popular C++ framework, to create graphical user interfaces (GUIs) in Python.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QWidget
from PyQt5.QtCore import Qt
class CustomWidget(QWidget):
def __init__(self):
super().__init__()
def mousePressEvent(self, event):
# Override the mousePressEvent to customize behavior
if event.button() == Qt.LeftButton:
print("Left mouse button clicked")
elif event.button() == Qt.RightButton:
print("Right mouse button clicked")
def main():
app = QApplication(sys.argv)
window = CustomWidget()
window.setWindowTitle("Custom Widget Example")
window.setGeometry(100, 100, 400, 300)
window.show()
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a custom widget class "CustomWidget" that inherits from "QWidget".
- Inside the CustomWidget class, we override the mousePressEvent event handler to customize its behavior. Depending on which mouse button was clicked (left or right), we print a message.
- In the main function, we create a "QApplication", instantiate our custom widget (CustomWidget), set the window's title and geometry, and show the window.
- When you run this program, it displays a window and start the application's event loop.
- When you click the left or right mouse button inside the widget, it prints a corresponding message to the console.
Output:
Left mouse button clicked Left mouse button clicked Right mouse button clicked Left mouse button clicked Right mouse button clicked![]()
Flowchart:
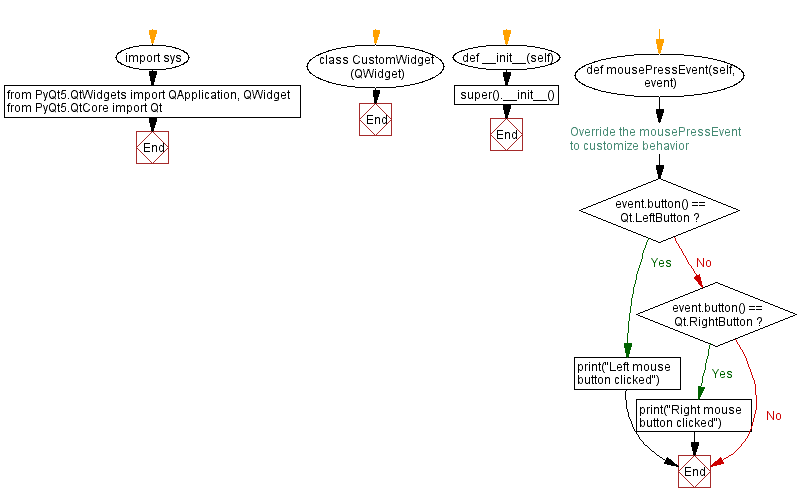
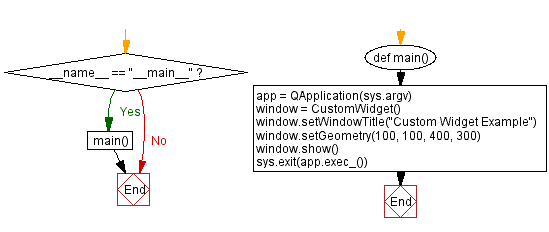
Go to:
Previous: Building a basic calculator with Python and PyQt.
Next: Creating a dialog box in PyQt.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.