Creating a custom widget in PyQt
Write a Python program using PyQt5 that asks users to change widget properties, such as color, font, or size. Observe how it affects the widget's appearance.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QVBoxLayout Class: This class is used to construct vertical box layout objects.
QWidget: The QWidget class is the base class of all user interface objects.
QColorDialog Class: The QColorDialog class provides a dialog widget for specifying colors.
QFontDialog Class: The QFontDialog class provides a dialog widget for selecting a font.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QPushButton, QVBoxLayout, QWidget, QColorDialog, QFontDialog
class WidgetPropertiesApp(QMainWindow):
def __init__(self):
super().__init__()
# Set the window properties (title and initial size)
self.setWindowTitle("Widget Properties")
self.setGeometry(100, 100, 400, 200) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
self.setCentralWidget(central_widget)
# Create a QPushButton
self.button = QPushButton("Click to Customize!")
# Create a vertical layout
layout = QVBoxLayout()
# Add the QPushButton to the layout
layout.addWidget(self.button)
# Set the layout for the central widget
central_widget.setLayout(layout)
# Connect button click events to customization methods
self.button.clicked.connect(self.customize_color)
self.button.clicked.connect(self.customize_font)
def customize_color(self):
# Allow the user to select a custom color for the button
color_dialog = QColorDialog.getColor()
if color_dialog.isValid():
self.button.setStyleSheet(f"background-color: {color_dialog.name()};")
def customize_font(self):
# Allow the user to select a custom font for the button text
font_dialog, ok = QFontDialog.getFont()
if ok:
self.button.setFont(font_dialog)
def main():
# Create a PyQt application
app = QApplication(sys.argv)
# Create an instance of the WidgetPropertiesApp class
window = WidgetPropertiesApp()
# Show the window
window.show()
# Run the application's event loop
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules.
- Create a custom "WidgetPropertiesApp" class that inherits from 'QMainWindow'.
- Inside the class, we create the main window and a 'QPushButton' that can be customized by the user.
- Create customization methods "customize_color()" and "customize_font()" that allow the user to select custom colors and fonts for the button.
- Connect the button's click events to these customization methods using 'clicked.connect'.
- The main function creates an instance of WidgetPropertiesApp, displays the window, and starts the application's event loop.
Output:
Flowchart:
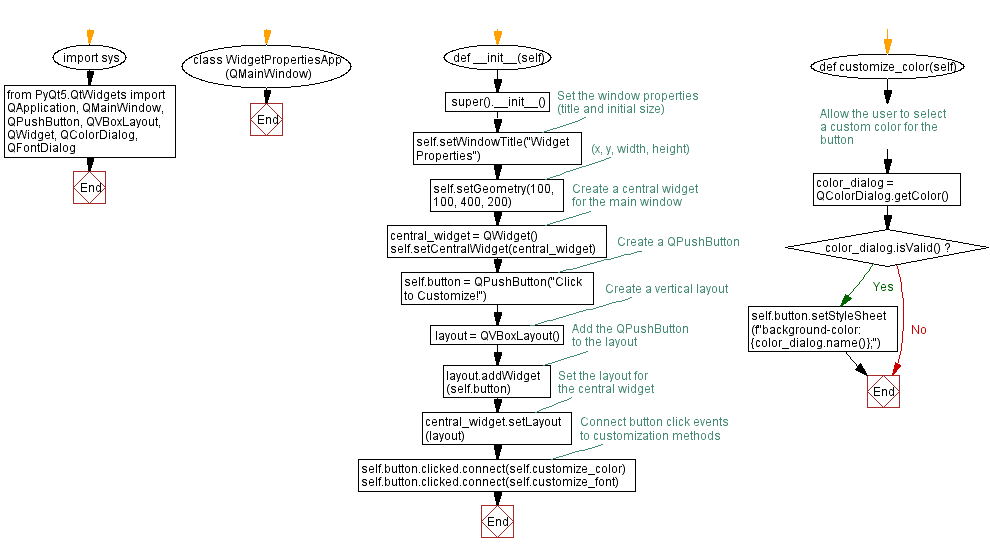
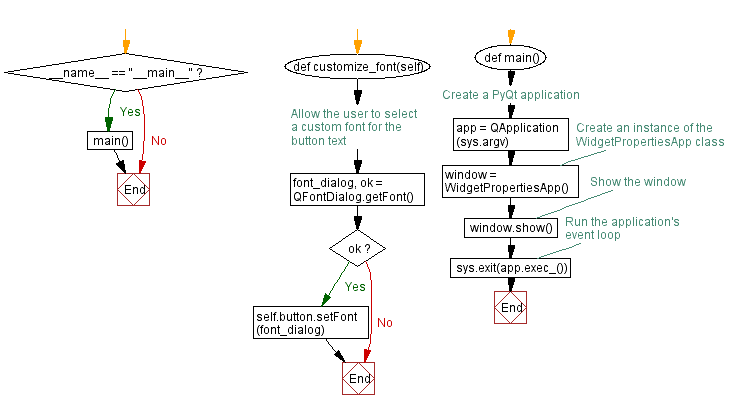
Go to:
Previous: Creating a custom widget with PyQt.
Next: Building a basic calculator with Python and PyQt.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.