Python PyQt5 basic application with widgets
Write a Python program to create a basic PyQt application that opens an empty window with a specified size and title. Add various widgets (e.g. label, push button) to the blank window. Widgets should display text or perform a simple action.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
QLabel Class: The QLabel widget provides a text or image display.
QPushButton: The push button, or command button, is perhaps the most commonly used widget in any graphical user interface. Push (click) a button to command the computer to perform some action, or to answer a question. Typical buttons are OK, Apply, Cancel, Close, Yes, No and Help.
QVBoxLayout Class: The QVBoxLayout class lines up widgets vertically.
QWidget: The QWidget class is the base class of all user interface objects.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow, QLabel, QPushButton, QVBoxLayout, QWidget
def main():
# Create a PyQt application
app = QApplication(sys.argv)
# Create a QMainWindow (main window)
main_window = QMainWindow()
# Set the window properties (title and initial size)
main_window.setWindowTitle("Basic PyQt Application")
main_window.setGeometry(100, 100, 400, 300) # (x, y, width, height)
# Create a central widget for the main window
central_widget = QWidget()
main_window.setCentralWidget(central_widget)
# Create widgets (QLabel and QPushButton)
label = QLabel("Example of PyQt label!")
button = QPushButton("Example of PyQt pushbutton!")
# Create a layout to arrange the widgets vertically
layout = QVBoxLayout()
layout.addWidget(label)
layout.addWidget(button)
# Set the layout for the central widget
central_widget.setLayout(layout)
# Show the window
main_window.show()
# Run the application's event loop
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a "QApplication" object to manage application control flow and settings.
- Create a "QMainWindow" object for the main application window and set its title and initial size.
- Create a central widget (a QWidget) to hold our other widgets.
- Create two widgets: a QLabel displaying "Example of PyQt label!" and a QPushButton with the label "Example of PyQt pushbutton!".
- Create a vertical layout (QVBoxLayout) and add 'QLabel' and 'QPushButton' to it.
- Set the layout for the central widget so that it arranges the widgets vertically.
- Finally, start the application's event loop with app.exec_() to handle user interactions and events.
Output:
Flowchart:
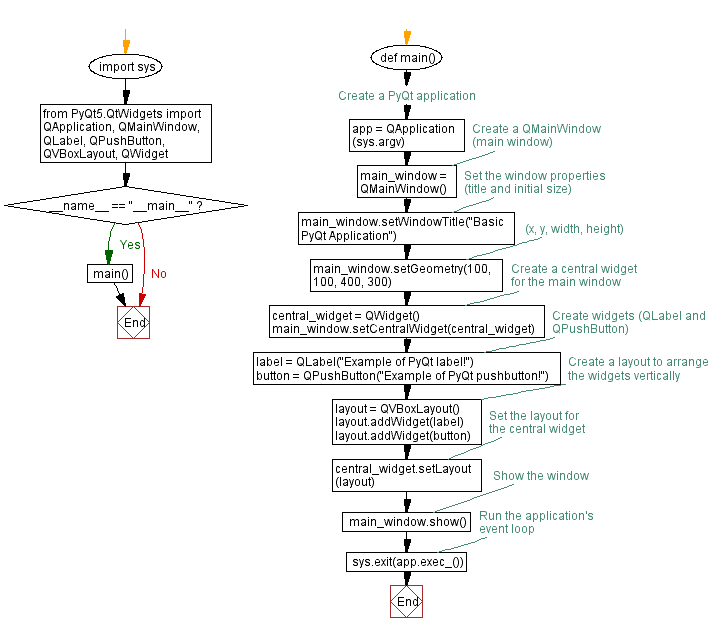
Go to:
Previous: Python PyQt5 blank window example.
Next: Python PyQt5 window with multiple widgets and layouts.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.