Python PyQt5 blank window example
Write a Python program to create a blank window using PyQt.
From doc.qt.io:
QApplication Class: The QApplication class manages the GUI application's control flow and main settings.
QMainWindow Class: The QMainWindow class provides a main application window.
Sample Solution:
Python Code:
import sys
from PyQt5.QtWidgets import QApplication, QMainWindow
def main():
# Create a PyQt application
app = QApplication(sys.argv)
# Create a QMainWindow (main window)
main_window = QMainWindow()
# Set the window properties (title and initial size)
main_window.setWindowTitle("Blank Window using PyQt")
main_window.setGeometry(100, 100, 400, 300) # (x, y, width, height)
# Show the window
main_window.show()
# Run the application's event loop
sys.exit(app.exec_())
if __name__ == "__main__":
main()
Explanation:
In the exercise above -
- Import the necessary modules from PyQt5.
- Create a 'QApplication' object, which manages the application's control flow and settings.
- Create a 'QMainWindow' object, which serves as our main application window.
- Set the window's title and initial size using the setWindowTitle and setGeometry methods.
- Show the window using "main_window.show()".
- Finally, start the application's event loop with app.exec_() to handle user interactions and events.
Output:
Flowchart:
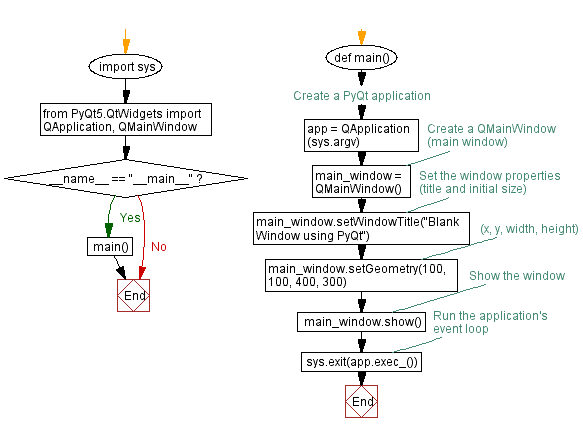
Go to:
Previous: Python PyQt Basic Home.
Next: Python PyQt5 basic application with widgets.
Python Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.