Python: Strange sort of list of numbers
Strange Sort: Alternating Min-Max
Write a Python program to find the following strange sort of list of numbers: the first element is the smallest, the second is the largest of the remaining, the third is the smallest of the remaining, the fourth is the smallest of the remaining, etc.
Input: [1, 3, 4, 5, 11] Output: [1, 11, 3, 5, 4] Input: [27, 3, 8, 5, 1, 31] Output: [1, 31, 3, 27, 5, 8] Input: [1, 2, 7, 3, 4, 5, 6] Output: [1, 7, 2, 6, 3, 5, 4]
Visual Presentation:
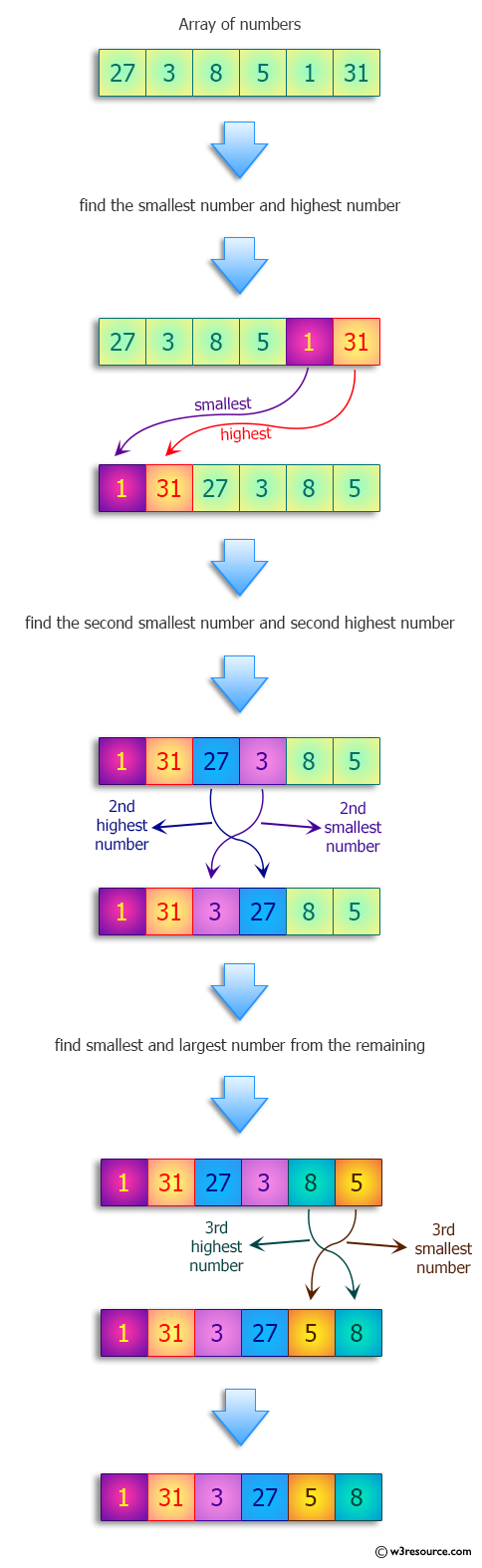
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Function to perform a strange sorting on a list of numbers
def test(nums):
# If the list has less than 2 elements, return the list as it is
if len(nums) < 2:
return nums
# Initialize an empty result list to store the sorted numbers
result = []
# Iterate through the first half of the list
for i in range(len(nums)//2):
# Append the minimum value to the result
result.append(min(nums))
# Remove the minimum value from the list
nums.remove(min(nums))
# Append the maximum value to the result
result.append(max(nums))
# Remove the maximum value from the list
nums.remove(max(nums))
# If there is one element left in the list, append it to the result
if len(nums) > 0:
result.append(nums[0])
# If the result list is still smaller than twice the length of the original list,
# extend it with the remaining elements from the original list
if len(result) < 2 * len(nums):
result.extend(nums[len(result) // 2 + 1:len(result) // 2 + 1 + len(nums) - len(result)])
# Return the final sorted list
return result
# Test cases with different lists of numbers
nums = [1, 3, 4, 5, 11]
print("Original list of numbers:")
print(nums)
print("Strange sort of list of said numbers:")
print(test(nums))
nums = [27, 3, 8, 5, 1, 31]
print("\nOriginal list of numbers:")
print(nums)
print("Strange sort of list of said numbers:")
print(test(nums))
nums = [1, 2, 7, 3, 4, 5, 6]
print("\nOriginal list of numbers:")
print(nums)
print("Strange sort of list of said numbers:")
print(test(nums))
Sample Output:
Original list of numbers: [1, 3, 4, 5, 11] Strange sort of list of said numbers: [1, 11, 3, 5, 4] Original list of numbers: [27, 3, 8, 5, 1, 31] Strange sort of list of said numbers: [1, 31, 3, 27, 5, 8] Original list of numbers: [1, 2, 7, 3, 4, 5, 6] Strange sort of list of said numbers: [1, 7, 2, 6, 3, 5, 4]
Flowchart:
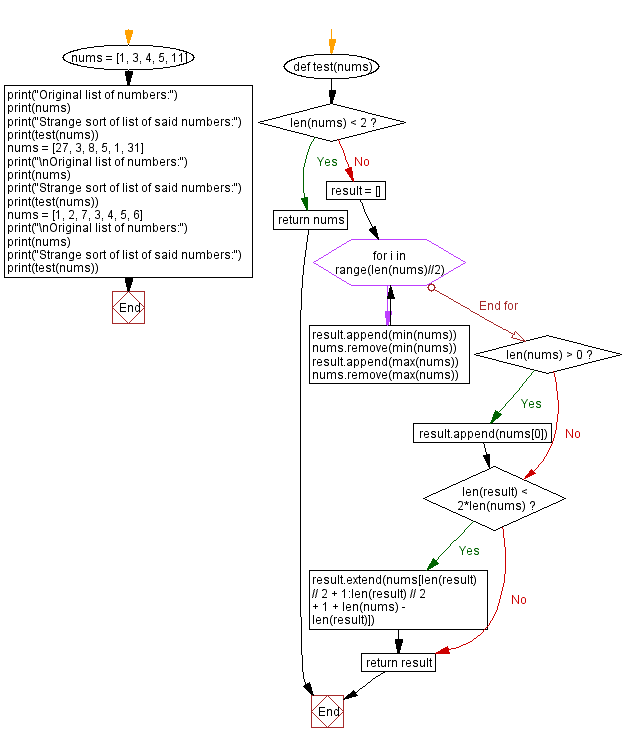
For more Practice: Solve these Related Problems:
- Write a Python program to rearrange a list so that the first element is the smallest, the second is the largest, the third is the next smallest, and so on.
- Write a Python program to implement an alternating min-max sort by repeatedly selecting and removing the minimum and maximum values.
- Write a Python program to construct a new list by alternatively appending the smallest and largest remaining elements from the original list.
- Write a Python program to use a greedy algorithm to generate an alternating sorted list from the input numbers.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Single digits in numbers sorted backwards and converted to English words.
Next: Compute the depth of groups of matched nested parentheses separated by parentheses.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics