Python: Find the closest palindrome
Python Programming Puzzles: Exercise-93 with Solution
Write a Python program to find the closest palindrome to a given string.
Input: cat Output: cac Input: madan Output: madam Input: radivider Output: radividar Input: madan Output: madam Input: abc Output: aba Input: racecbr Output: racecar
Sample Solution:
Python Code:
# Define a function named 'test' that finds the closest palindrome of a given string 's'
def test(s):
# Initialize a variable 'odd' to count the number of differing characters between mirrored positions
odd = 0
# Iterate through the characters of the string along with their indices
for i, c in enumerate(s):
# Check if the character at the current position is not equal to its mirrored position
if c != s[~i]:
# Increment the 'odd' count
odd += 1
# Check if the count of differing characters is an odd number
if odd % 2 == 1:
# Calculate the half of the 'odd' count
half = odd // 2
# Construct a palindrome by taking characters from the original string and its mirrored positions
pal = "".join((s[i] if i < half else s[~i] for i in range(len(s))))
# Return the closest palindrome
return pal
else:
# Calculate the half of the 'odd' count
half = odd // 2
# Construct a palindrome by taking characters from the original string and its mirrored positions
pal = "".join((s[i] if i <= half else s[~i] for i in range(len(s))))
# Return the closest palindrome
return pal
# Example 1
s1 = "cat"
print("Original string:", s1)
print("Closest palindrome of the said string:")
print(test(s1))
# Example 2
s2 = "madan"
print("\nOriginal string:", s2)
print("Closest palindrome of the said string:")
print(test(s2))
# Example 3
s3 = "radivider"
print("Original string:", s3)
print("Closest palindrome of the said string:")
print(test(s3))
# Example 4
s4 = "madan"
print("\nOriginal string:", s4)
print("Closest palindrome of the said string:")
print(test(s4))
# Example 5
s5 = "abc"
print("Original string:", s5)
print("Closest palindrome of the said string:")
print(test(s5))
# Example 6
s6 = "racecbr"
print("\nOriginal string:", s6)
print("Closest palindrome of the said string:")
print(test(s6))
Sample Output:
Original string: cat Closest palindrome of the said string: cac Original string: madan Closest palindrome of the said string: madam Original string: radivider Closest palindrome of the said string: radividar Original string: madan Closest palindrome of the said string: madam Original string: abc Closest palindrome of the said string: aba Original string: racecbr Closest palindrome of the said string: racecar
Flowchart:
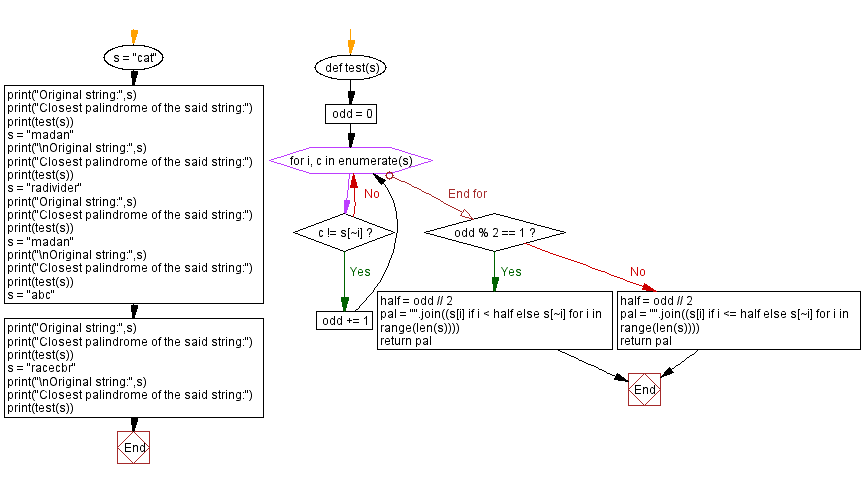
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Start with a list of integers, keep every other element in place and otherwise sort the list.
Next: Separate parentheses groups.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/puzzles/python-programming-puzzles-93.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics