Python: Find all n-digit integers that start or end with 2
Python Programming Puzzles: Exercise-91 with Solution
Write a Python program to find all n-digit integers that start or end with 2.
Input: 1 Output: [2] Input: 2 Output: [12, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 32, 42, 52, 62, 72, 82, 92] Input: 3 Output: [102, 112, 122, 132, 142, 152, 162, 172, 182, 192, 200, 201, 202, 203, 204, 205, 206, 207, 208, 209, 210, 211, 212, 213, 214, 215, 216, 217, 218, 219, 220, 221, 222, 223, 224, 225, 226, 227, 228, 229, 230, 231, 232, 233, 234, 235, 236, 237, 238, 239, 240, 241, 242, 243, 244, 245, 246, 247, 248, 249, 250, 251, 252, 253, 254, 255, 256, 257, 258, 259, 260, 261, 262, 263, 264, 265, 266, 267, 268, 269, 270, 271, 272, 273, 274, 275, 276, 277, 278, 279, 280, 281, 282, 283, 284, 285, 286, 287, 288, 289, 290, 291, 292, 293, 294, 295, 296, 297, 298, 299, 302, 312, 322, 332, 342, 352, 362, 372, 382, 392, 402, 412, 422, 432, 442, 452, 462, 472, 482, 492, 502, 512, 522, 532, 542, 552, 562, 572, 582, 592, 602, 612, 622, 632, 642, 652, 662, 672, 682, 692, 702, 712, 722, 732, 742, 752, 762, 772, 782, 792, 802, 812, 822, 832, 842, 852, 862, 872, 882, 892, 902, 912, 922, 932, 942, 952, 962, 972, 982, 992]
Visual Presentation:
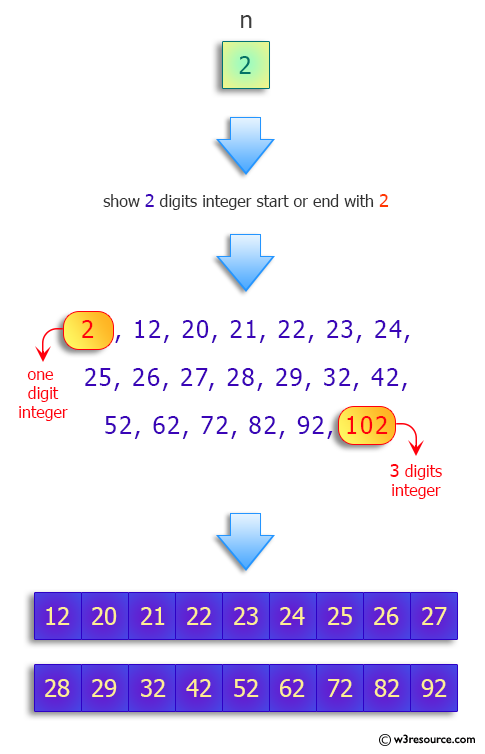
Sample Solution:
Python Code:
# Define a function named 'test' that takes an integer 'n' as a parameter
def test(n):
# Initialize an empty list to store the results
ans = []
# Iterate through all n-digit integers
for i in range(10 ** (n - 1), 10 ** n):
# Assert that the length of the current integer is equal to 'n'
assert len(str(i)) == n
# Check if the integer starts or ends with '2'
if str(i).startswith("2") or str(i).endswith("2"):
ans.append(i)
# Return the list of integers that meet the specified conditions
return ans
# Example 1
n1 = 1
print("Number:", n1)
print("All", n1, "- digit integers that start or end with 2:")
print(test(n1))
# Example 2
n2 = 2
print("\nNumber:", n2)
print("All", n2, "- digit integers that start or end with 2:")
print(test(n2))
# Example 3
n3 = 3
print("\nNumber:", n3)
print("All", n3, "- digit integers that start or end with 2:")
print(test(n3))
Sample Output:
Number: 1 All 1 - digit integers that start or end with 2: [2] Number: 2 All 2 - digit integers that start or end with 2: [12, 20, 21, 22, 23, 24, 25, 26, 27, 28, 29, 32, 42, 52, 62, 72, 82, 92] Number: 3 All 3 - digit integers that start or end with 2: [102, 112, 122, 132, 142, 152, 162, 172, 182, 192, 200, 201, 202, 203, 204, 205, 206, 207, 208, 209, 210, 211, 212, 213, 214, 215, 216, 217, 218, 219, 220, 221, 222, 223, 224, 225, 226, 227, 228, 229, 230, 231, 232, 233, 234, 235, 236, 237, 238, 239, 240, 241, 242, 243, 244, 245, 246, 247, 248, 249, 250, 251, 252, 253, 254, 255, 256, 257, 258, 259, 260, 261, 262, 263, 264, 265, 266, 267, 268, 269, 270, 271, 272, 273, 274, 275, 276, 277, 278, 279, 280, 281, 282, 283, 284, 285, 286, 287, 288, 289, 290, 291, 292, 293, 294, 295, 296, 297, 298, 299, 302, 312, 322, 332, 342, 352, 362, 372, 382, 392, 402, 412, 422, 432, 442, 452, 462, 472, 482, 492, 502, 512, 522, 532, 542, 552, 562, 572, 582, 592, 602, 612, 622, 632, 642, 652, 662, 672, 682, 692, 702, 712, 722, 732, 742, 752, 762, 772, 782, 792, 802, 812, 822, 832, 842, 852, 862, 872, 882, 892, 902, 912, 922, 932, 942, 952, 962, 972, 982, 992]
Flowchart:
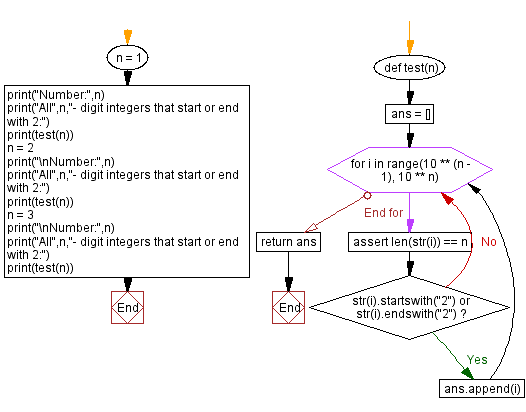
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Each triple of eaten, need, stock return a pair of total appetite and remaining.
Next: Start with a list of integers, keep every other element in place and otherwise sort the list.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics