Python: Each triple of eaten, need, stock return a pair of total appetite and remaining
Appetite and Stock Calculation
For each triple of eaten, need, and stock, write a Python program to get a pair of total appetite and remaining.
Input: [[2, 5, 6], [3, 9, 22]] Output: [[7, 1], [12, 13]] Input: [[2, 3, 18], [4, 9, 2], [2, 5, 7], [3, 8, 12], [4, 9, 106]] Output: [[5, 15], [6, 0], [7, 2], [11, 4], [13, 97]] Input: [[1, 2, 3], [4, 5, 6]] Output: [[3, 1], [9, 1]]
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of triples as parameter 'nums'
def test(nums):
# Return a list of lists, where each inner list contains the total appetite and remaining items
return [[a + min(b, c), max(0, c - b)] for a, b, c in nums]
# Example 1
nums1 = [[2, 5, 6], [3, 9, 22]]
print("Original list (triple) of lists:")
print(nums1)
print("Each triple of eaten, need, stock return a pair of total appetite and remaining:")
print(test(nums1))
# Example 2
nums2 = [[2, 3, 18], [4, 9, 2], [2, 5, 7], [3, 8, 12], [4, 9, 106]]
print("\nOriginal list (triple) of lists:")
print(nums2)
print("Each triple of eaten, need, stock return a pair of total appetite and remaining:")
print(test(nums2))
# Example 3
nums3 = [[1, 2, 3], [4, 5, 6]]
print("\nOriginal list (triple) of lists:")
print(nums3)
print("Each triple of eaten, need, stock return a pair of total appetite and remaining:")
print(test(nums3))
Sample Output:
Original list (triple) of lists: [[2, 5, 6], [3, 9, 22]] Each triple of eaten, need, stock return a pair of total appetite and remaining: [[7, 1], [12, 13]] Original list (triple) of lists: [[2, 3, 18], [4, 9, 2], [2, 5, 7], [3, 8, 12], [4, 9, 106]] Each triple of eaten, need, stock return a pair of total appetite and remaining: [[5, 15], [6, 0], [7, 2], [11, 4], [13, 97]] Original list (triple) of lists: [[1, 2, 3], [4, 5, 6]] Each triple of eaten, need, stock return a pair of total appetite and remaining: [[3, 1], [9, 1]]
Flowchart:
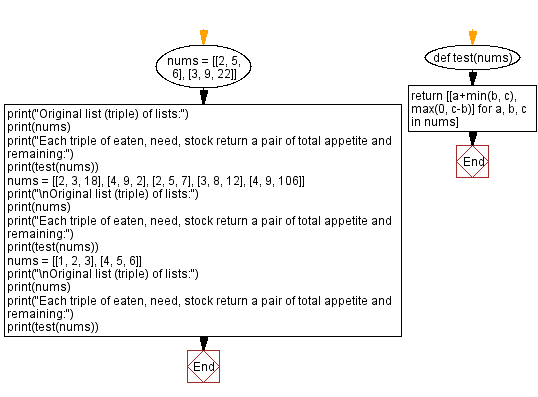
For more Practice: Solve these Related Problems:
- Write a Python program to process a list of triples [eaten, need, stock] and return a list of pairs [total appetite, remaining stock].
- Write a Python program to iterate over a list of three-element lists and calculate the sum of the first two elements, then subtract it from the third.
- Write a Python program to use list comprehension to compute, for each triple, the pair (sum of eaten and need, stock minus that sum).
- Write a Python program to implement a function that takes a triple and returns the calculated appetite and remaining stock as a pair.
Go to:
Previous: Find all integers that are the product of exactly three primes.
Next: Find all n-digit integers that start or end with 2.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.