Python: List integers containing exactly three distinct values, such that no integer repeats twice consecutively
Python Programming Puzzles: Exercise-9 with Solution
Write a Python program to find a list of integers containing exactly four distinct values, such that no integer repeats twice consecutively among the first twenty entries.
Note: The list needs to have length greater than ten.)
Input: [1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4] Output: True Input: [1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3] Output: False Input: [1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3] Output: False
Visual Presentation:
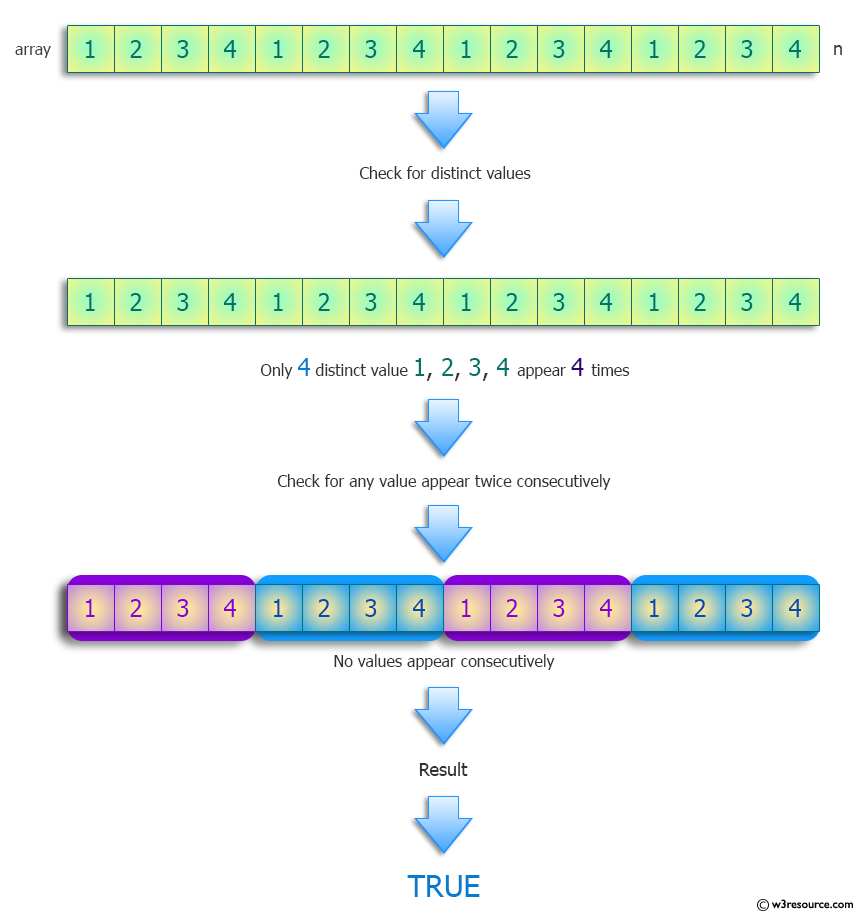
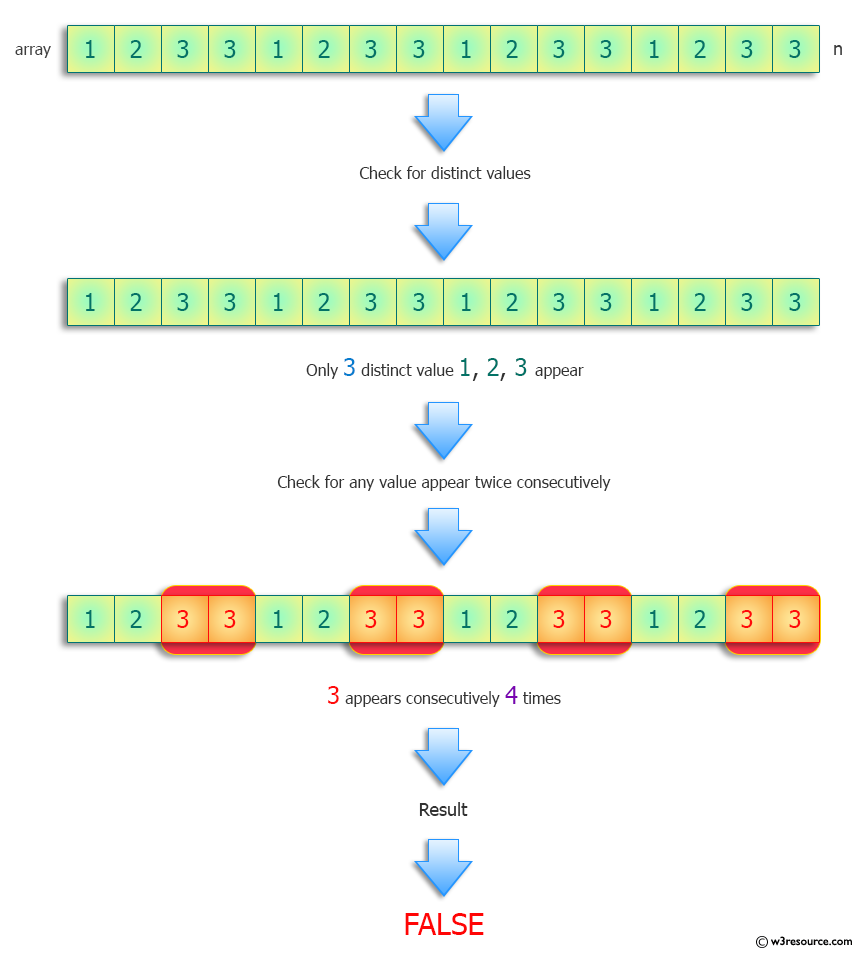
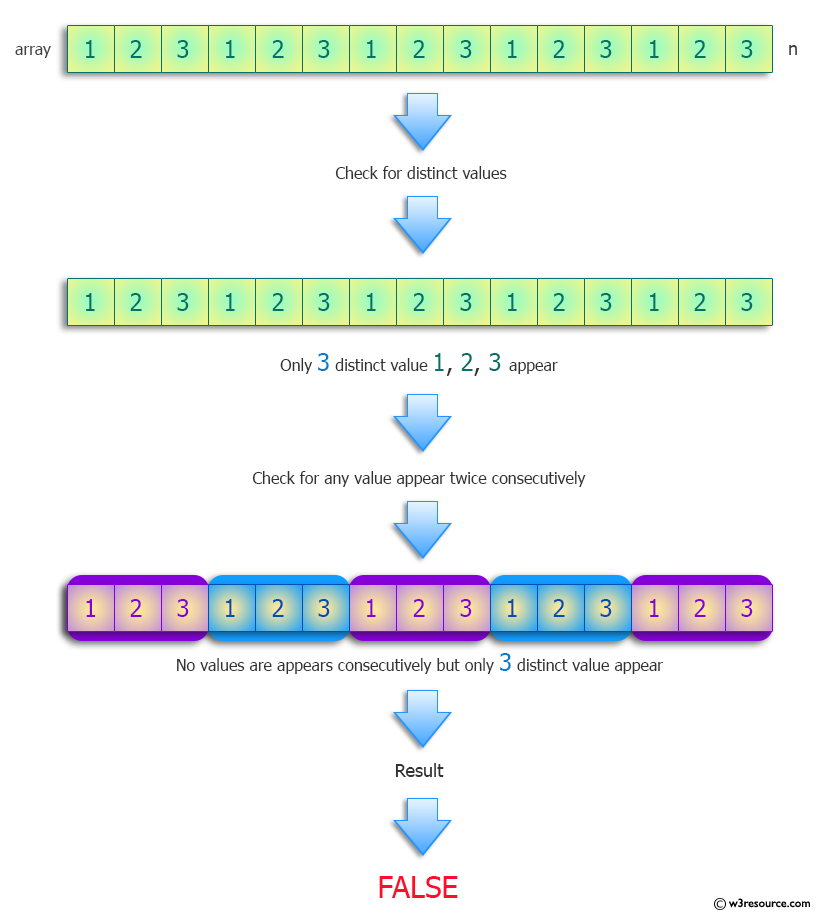
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of integers 'nums' as input
def test(nums):
# Check if no integer in 'nums' repeats consecutively and if there are exactly four distinct values in 'nums'
return all([nums[i] != nums[i + 1] for i in range(len(nums) - 1)]) and len(set(nums)) == 4
# Create a list of integers 'nums' with specific elements
nums = [1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4]
# Print the original list
print("Original list:")
print(nums)
# Print a message indicating the condition being checked on the list
print("Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Create a different list of integers 'nums' with specific elements
nums = [1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3]
# Print the original list
print("\nOriginal list:")
print(nums)
# Print a message indicating the condition being checked on the list
print("Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively:")
# Print the result of the test function applied to the modified 'nums' list
print(test(nums))
# Create another list of integers 'nums' with specific elements
nums = [1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3]
# Print the original list
print("\nOriginal list:")
print(nums)
# Print a message indicating the condition being checked on the list
print("Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively:")
# Print the result of the test function applied to the modified 'nums' list
print(test(nums))
Sample Output:
Original list: [1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4, 1, 2, 3, 4] Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively: True Original list: [1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3, 1, 2, 3, 3] Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively: False Original list: [1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3, 1, 2, 3] Check said list of integers containing exactly four distinct values, such that no integer repeats twice consecutively: False
Flowchart:
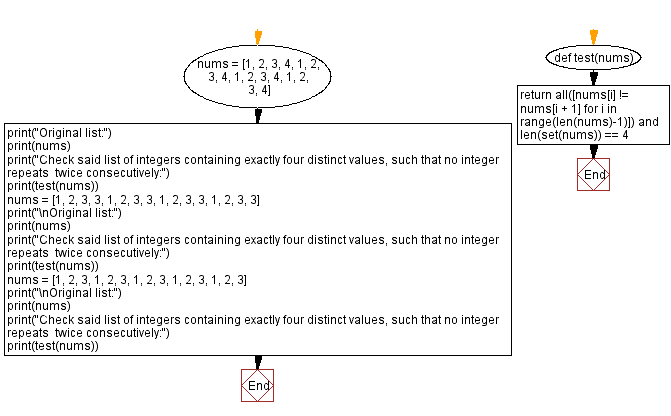
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Split a string of words separated by commas and spaces into 2 lists: words and separators.
Next: Separate Parentheses Groups Perfectly.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/puzzles/python-programming-puzzles-9.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics