Python: Find an integer with the given number of even and odd digits
Integer with Specified Even and Odd Digits
Write a Python program to find an integer (n >= 0) with the given number of even and odd digits.
Input: Number of even digits: 2 ,Number of odd digits: 3 Output: 22333 Input: Number of even digits: 4 ,Number of odd digits: 7 Output: 22223333333
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes two parameters 'evens' and 'odds'
def test(evens, odds):
# Generate an integer (>= 0) with the given number of even and odd digits
return int(evens * "2" + odds * "3")
# Example 1
evens1 = 2
odds1 = 3
print("Number of even digits:", evens1, ", Number of odd digits:", odds1)
print("Integer (>= 0) with the given number of even and odd digits:")
print(test(evens1, odds1))
# Example 2
evens2 = 4
odds2 = 7
print("\nNumber of even digits:", evens2, ", Number of odd digits:", odds2)
print("Integer (>= 0) with the given number of even and odd digits:")
print(test(evens2, odds2))
Sample Output:
Number of even digits: 2 ,Number of odd digits: 3 Integer(>= 0) with the given number of even and odd digits: 22333 Number of even digits: 4 ,Number of odd digits: 7 Integer(>= 0) with the given number of even and odd digits: 22223333333
Flowchart:
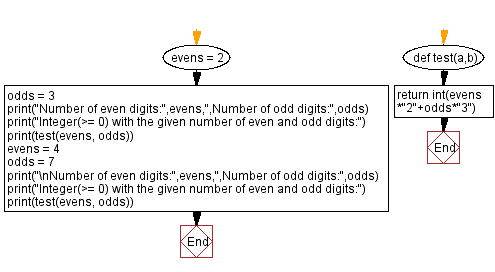
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find a valid substring of s that contains matching brackets, at least one of which is nested.
Next: Find all integers that are the product of exactly three primes.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics