Python: Find a valid substring of s that contains matching brackets, at least one of which is nested
Valid Substring with Nested Brackets
Write a Python program to find a valid substring of a given string that contains matching brackets, at least one of which is nested.
Input: ]][][[]]] Output: [[]] Input: ]]]]]]]]]]]]]]]]][][][][]]]]]]]]]]][[[][[][[[[[][][][]][[[[[[[[[[[[[[[[[[ Output: [[][][][]]
Visual Presentation:
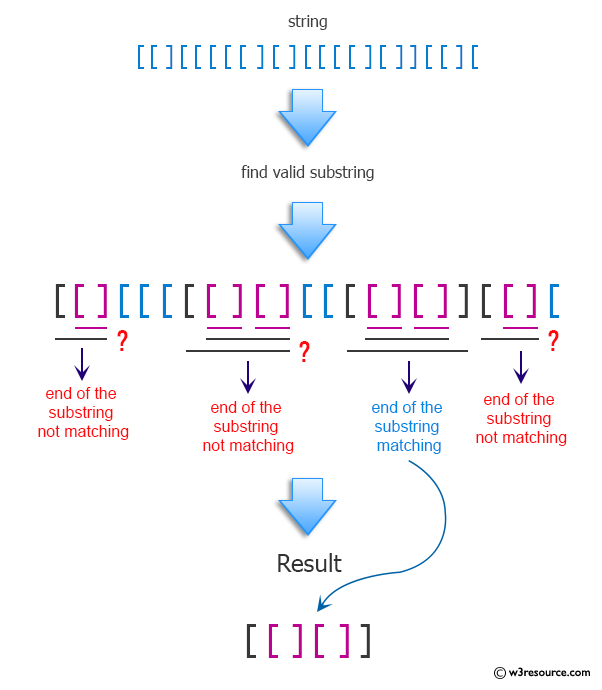
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 's' as input
def test(s):
import re
# Use regular expression to search for a valid substring with matching brackets
return re.search(r"\[(\[\])+\]", s).group(0)
# Example 1
brackets1 = "]][][[]]]"
print("Original List of strings:", brackets1)
print("Find a valid substring of the said string that contains matching brackets, at least one of which is nested:")
print(test(brackets1))
# Example 2
brackets2 = "]]]]]]]]]]]]]]]]][][][][]]]]]]]]]]][[[][[][[[[[][][][]][[[[[[[[[[[[[[[[[["
print("\nOriginal List of strings:", brackets2)
print("\nFind a valid substring of the said string that contains matching brackets, at least one of which is nested:")
print(test(brackets2))
Sample Output:
Original List of strings: ]][][[]]] Find a valid substring of the said string that contains matching brackets, at least one of which is nested: [[]] Original List of strings: ]]]]]]]]]]]]]]]]][][][][]]]]]]]]]]][[[][[][[[[[][][][]][[[[[[[[[[[[[[[[[[ Find a valid substring of the said string that contains matching brackets, at least one of which is nested: [[][][][]]
Flowchart:
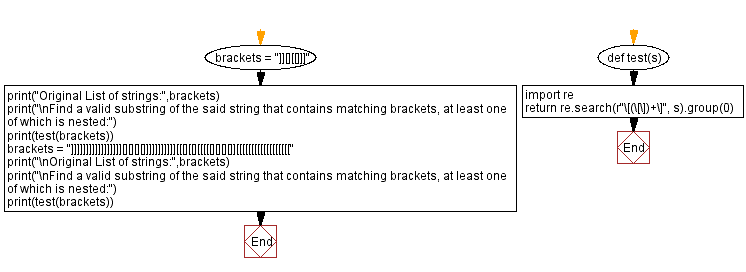
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the vowels from each of the original texts (y counts as a vowel at the end of the word).
Next: Find an integer with the given number of even and odd digits.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics