Python: Calculate the average of the numbers a through b rounded to nearest integer, in binary
Average in Binary of a Range
Write a Python program to calculate the average of the numbers a through b (b not included) rounded to the nearest integer, in binary (or -1 if there are no such numbers).
Input: 4 , 7 Output: 0b101 Input: 11 , 19 Output: 0b1110
Visual Presentation:
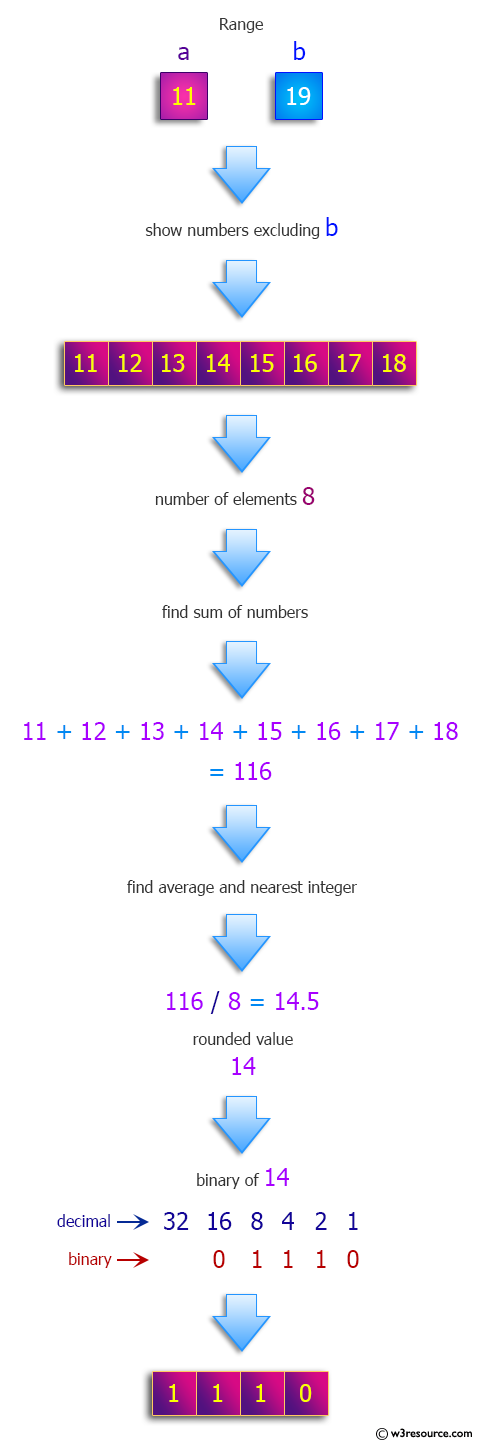
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes two integer parameters, 'a' and 'b'
def test(a, b):
# Create a range of integers from 'a' to 'b' (exclusive)
r = range(a, b)
# Check if the range is empty
if len(r) == 0:
return "-1" # Return "-1" if the range is empty
# Calculate the average of the numbers in the range, round to the nearest integer,
# and convert the result to binary representation
return bin(round(sum(r) / len(r)))
# Example 1
a1 = 4
b1 = 7
print("Range:", a1, ",", b1)
print("Average of the numbers", a1, "through", b1, "rounded to the nearest integer, in binary:")
print(test(a1, b1))
# Example 2
a2 = 11
b2 = 19
print("\nRange:", a2, ",", b2)
print("Average of the numbers", a2, "through", b2, "rounded to the nearest integer, in binary:")
print(test(a2, b2))
Sample Output:
Range: 4 , 7 Average of the numbers 4 through 7 rounded to nearest integer, in binary: 0b101 Range: 11 , 19 Average of the numbers 11 through 19 rounded to nearest integer, in binary: 0b1110
Flowchart:
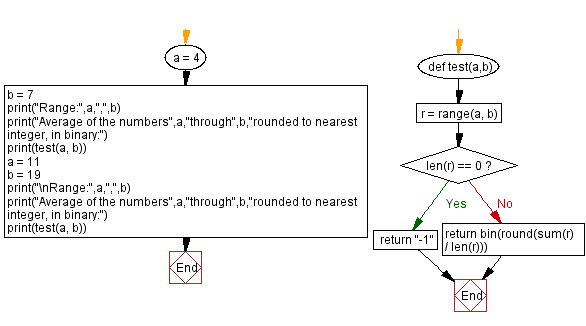
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Round each float in a list of numbers up to the next integer and return the running total of the integer squares.
Next: Find the sublist of numbers with only odd digits in increasing order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics