Python: Round each float in a list of numbers up to the next integer and return the running total of the integer squares
Running Total of Integer Squares
Write a Python program to round each float in a given list of numbers up to the next integer and return the running total of the integer squares.
Input: [2.6, 3.5, 6.7, 2.3, 5.6] Output: [9, 25, 74, 83, 119] Input: [301.1, 401.4, -23.1, 13554122.0, 10201.0101, 10000000.0] Output: [91204, 252808, 253337, 183714223444221, 183714327525025, 283714327525025]
Visual Presentation:
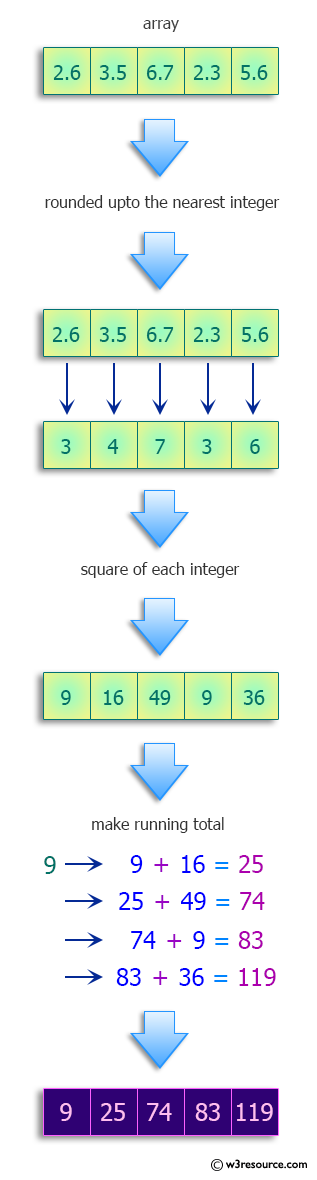
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Import the 'ceil' function from the 'math' module
from math import ceil
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Initialize an empty list to store the running total of integer squares
running_squares = []
# Initialize a variable 'tot' to store the running total
tot = 0
# Iterate over each value 'v' in the input list 'nums'
for v in nums:
# Add the square of the ceiling of 'v' to the running total
tot += ceil(v) ** 2
# Append the current running total to the 'running_squares' list
running_squares.append(tot)
# Return the list of running totals
return running_squares
# Example 1
nums1 = [2.6, 3.5, 6.7, 2.3, 5.6]
print("List of numbers:", nums1)
print("Round each float of the said list up to the next integer and return the running total of the integer squares:")
print(test(nums1))
# Example 2
nums2 = [301.1, 401.4, -23.1, 13554122.0, 10201.0101, 10000000.0]
print("\nList of numbers:", nums2)
print("Round each float of the said list up to the next integer and return the running total of the integer squares:")
print(test(nums2))
Sample Output:
List of numbers: [2.6, 3.5, 6.7, 2.3, 5.6] Round each float of the said list up to the next integer and return the running total of the integer squares: [9, 25, 74, 83, 119] List of numbers: [301.1, 401.4, -23.1, 13554122.0, 10201.0101, 10000000.0] Round each float of the said list up to the next integer and return the running total of the integer squares: [91204, 252808, 253337, 183714223444221, 183714327525025, 283714327525025]
Flowchart:
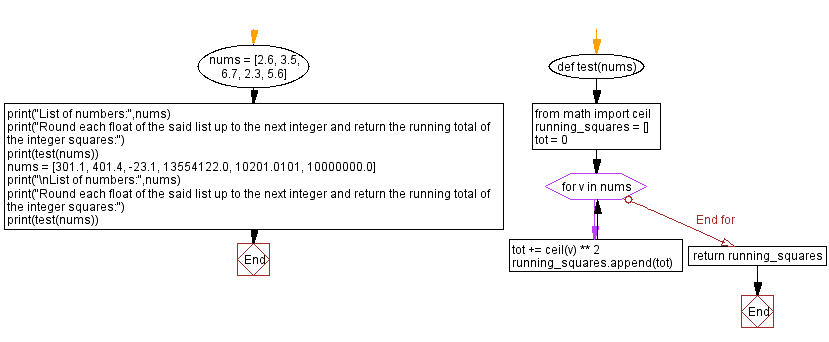
For more Practice: Solve these Related Problems:
- Write a Python program to round up each float in a list to the next integer, square it, and compute the running total of these squares.
- Write a Python program to use math.ceil() on each element of a list, square the result, and generate a cumulative sum list.
- Write a Python program to iterate over a list of floats, round them up, square them, and produce a list of running totals.
- Write a Python program to use list comprehension to create a new list of squared ceiling values, then compute their cumulative sum.
Go to:
Previous: Find the largest negative and smallest positive numbers.
Next: Calculate the average of the numbers a through b rounded to nearest integer, in binary.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.