Python: Find a string consisting of space-separated characters with given counts
Space-Separated Characters with Counts
Write a Python program to find a string consisting of space-separated characters with given counts.
Input: {'f': 1, 'o': 2} Output: f o o Input: {'a': 1, 'b': 1, 'c': 1} Output: a b c
Visual Presentation:
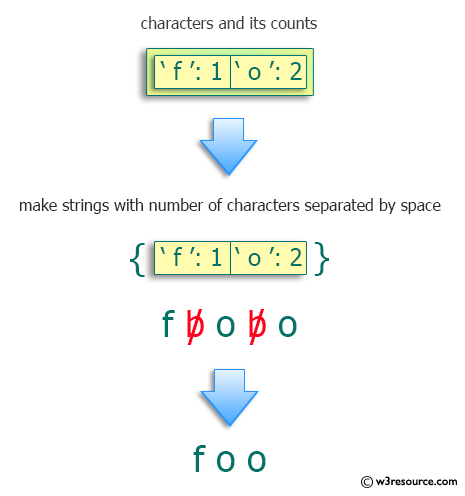
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a dictionary of character counts as input
def test(counts):
# Use a nested loop to generate a string with characters repeated according to their counts
return " ".join(c for c, i in counts.items() for _ in range(i))
# Example 1
strs1 = {"f": 1, "o": 2}
print("Original string:", strs1)
print("String consisting of space-separated characters with given counts:")
print(test(strs1))
# Example 2
strs2 = {"a": 1, "b": 1, "c": 1}
print("\nOriginal string:", strs2)
print("String consisting of space-separated characters with given counts:")
print(test(strs2))
Sample Output:
Original string: {'f': 1, 'o': 2} String consisting of space-separated characters with given counts: f o o Original string: {'a': 1, 'b': 1, 'c': 1} String consisting of space-separated characters with given counts: a b c
Flowchart:
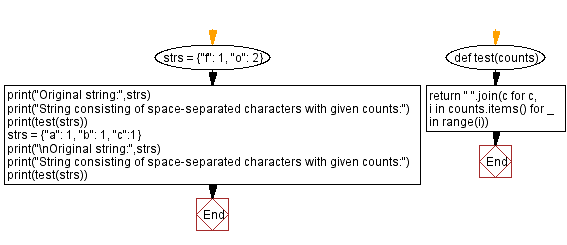
For more Practice: Solve these Related Problems:
- Write a Python program to convert a dictionary mapping characters to counts into a space-separated string where each character is repeated as per its count.
- Write a Python program to expand a dictionary of character frequencies into a list of characters and then join them with spaces.
- Write a Python program to iterate over a dictionary and construct a string with each key repeated by its frequency, separated by spaces.
- Write a Python program to use list comprehension to produce a string from a dictionary of counts, with each character occurring the specified number of times.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find a string contains a vowel between two consonants, in a given string.
Next: Reorder numbers in increasing/decreasing order based on whether the first plus last element is even/odd.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics