Python: Find the indices of three numbers that sum to 0 in a list
Indices of Three Numbers Summing to Zero
Write a Python program to find the indices of three numbers that sum to 0 in a given list of numbers.
Input: [12, -7, 3, -89, 14, 4, -78, -1, 2, 7] Output: [1, 2, 5] Input: [1, 2, 3, 4, 5, 6, -7] Output: [2, 3, 6]
Visual Presentation:
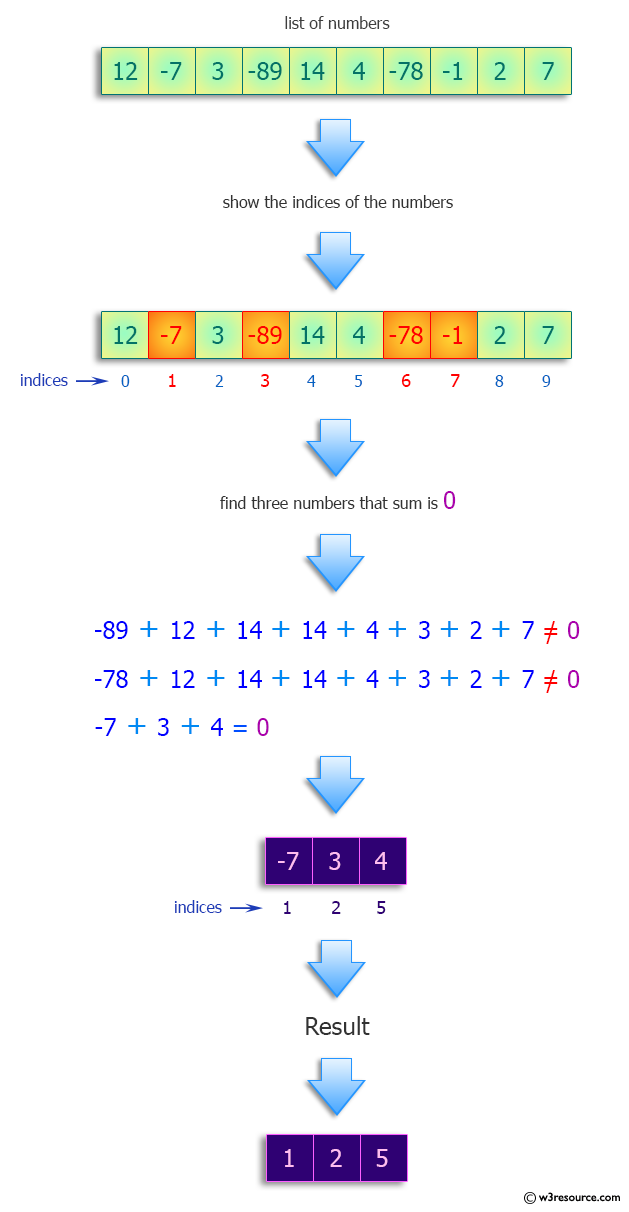
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
inv = {n: i for i, n in enumerate(nums)} # Create a dictionary to store the indices of numbers
# Note that later duplicates will override earlier entries
# Iterate through the indices and numbers in 'nums'
for i, n in enumerate(nums):
if inv[n] == i:
del inv[n] # Remove the entry if it corresponds to the current index
# Check if there is a pair of indices (j, k) such that nums[j] + nums[k] + n = 0
if any((-m - n) in inv for m in nums[:i]):
# If a solution is found, get the indices (j, m) where nums[j] + m = -n
j, m = next((j, m) for j, m in enumerate(nums) if (-m - n) in inv)
# Get the index k for nums[k] = -m - n
k = inv[-m - n]
return sorted([i, j, k]) # Return the sorted list of indices
# Example 1
nums1 = [12, -7, 3, -89, 14, 4, -78, -1, 2, 7]
print("List of numbers:", nums1)
print("Indices of three numbers that sum to 0 in the said list:")
print(test(nums1))
# Example 2
nums2 = [1, 2, 3, 4, 5, 6, -7]
print("\nList of numbers:", nums2)
print("Indices of three numbers that sum to 0 in the said list:")
print(test(nums2))
Sample Output:
List of numbers: [12, -7, 3, -89, 14, 4, -78, -1, 2, 7] Indices of three numbers that sum to 0 in the said list: [1, 2, 5] List of numbers: [1, 2, 3, 4, 5, 6, -7] Indices of three numbers that sum to 0 in the said list: [2, 3, 6]
Flowchart:
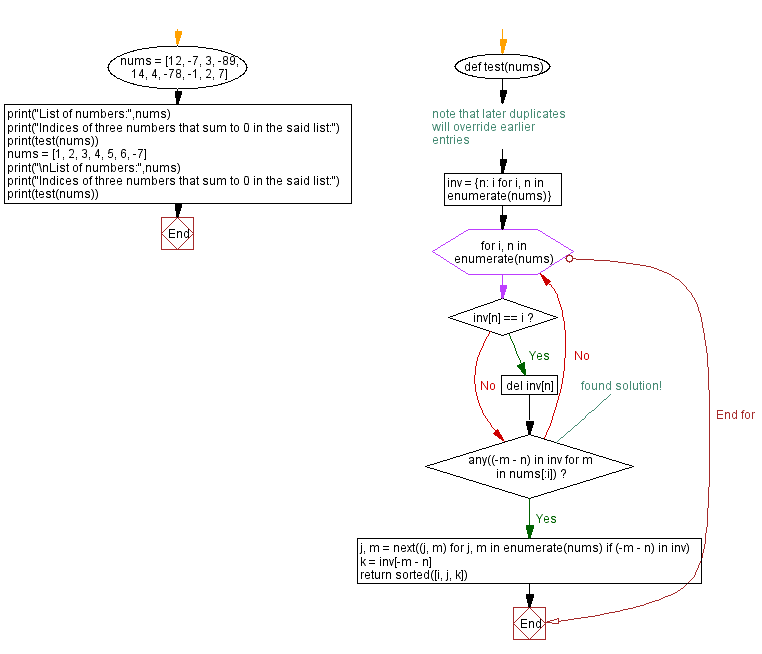
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Inject a number in between each pair of adjacent numbers in a list of numbers.
Next: Find a string contains a vowel between two consonants, in a given string.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics