Python: Find the number which when appended to the list makes the total 0
Find Missing Number for Zero Total
Write a Python program to find the number which when appended to the list makes the total 0.
Input: [1, 2, 3, 4, 5] Output: -15 Input: [-1, -2, -3, -4, 5] Output: 5 Input: [10, 42, 17, 9, 1315182, 184, 102, 29, 15, 39, 755] Output: -1316384
Visual Presentation:
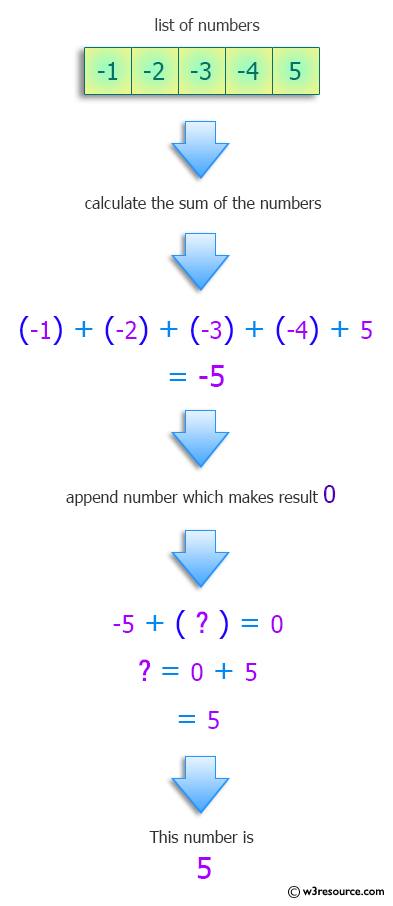
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers as input
def test(nums):
# Create a set 'dset' from the input list to remove duplicates
dset = set(nums)
# Initialize 'result' with the sum of the original list 'nums'
result = sum(nums)
# Calculate the absolute difference between the minimum value in 'nums' and the sum of the symmetric difference between 'dset' and 'nums'
dmin = abs(min(nums) - sum(dset ^ set(nums)))
# Iterate over the symmetric difference between 'dset' and 'nums'
for d in dset ^ set(nums):
# Create a copy of 'nums' and append the current value 'd'
dcopy = list(nums)
dcopy.append(d)
# Calculate the sum of the modified list
ds = sum(dcopy)
# Check if the absolute value of the negation of the sum is less than 'dmin'
if 0 - ds < dmin:
result = ds
dmin = abs(ds)
# Check if the absolute value of the negation of the sum is equal to 'dmin'
elif 0 - ds == dmin:
# Update 'result' with the minimum value between the current 'result' and the calculated sum 'ds'
result = min(result, ds)
# Multiply the final result by -1 and return it
return result * (-1)
# Set a list of numbers
nums = [1, 2, 3, 4, 5]
# Print a message indicating the original list of numbers
print("Original list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the number that, when appended to the list, makes the total 0
print("Number which when appended to the list makes the total 0:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
# Set another list of numbers
nums = [-1, -2, -3, -4, 5]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the number that, when appended to the list, makes the total 0
print("Number which when appended to the list makes the total 0:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
# Set yet another list of numbers
nums = [10, 42, 17, 9, 1315182, 184, 102, 29, 15, 39, 755]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the number that, when appended to the list, makes the total 0
print("Number which when appended to the list makes the total 0:")
# Print the result of the 'test' function applied to the numbers
print(test(nums))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5] Number which when appended to the list makes the total 0: -15 Original list of numbers: [-1, -2, -3, -4, 5] Number which when appended to the list makes the total 0: 5 Original list of numbers: [10, 42, 17, 9, 1315182, 184, 102, 29, 15, 39, 755] Number which when appended to the list makes the total 0: -1316384
Flowchart:
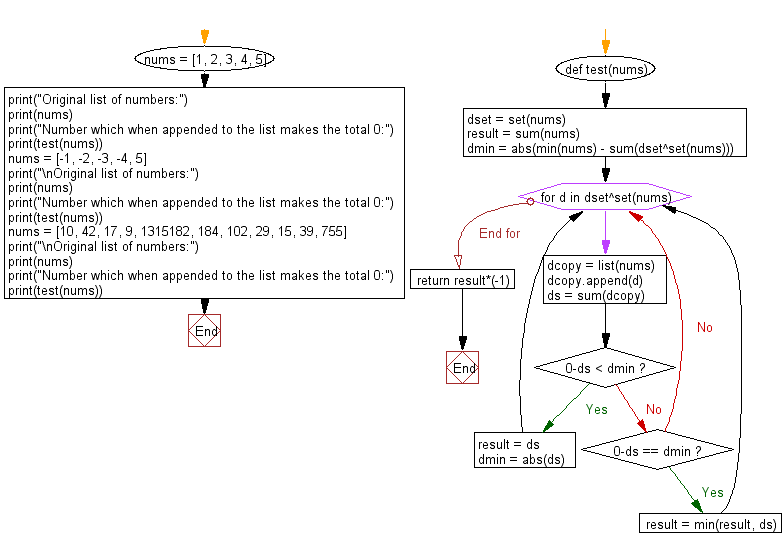
For more Practice: Solve these Related Problems:
- Write a Python program to compute the missing number which, when added to a list of integers, makes the total sum zero.
- Write a Python program to determine the additive inverse of the sum of a list and return it as the missing number.
- Write a Python program to use the sum() function to calculate the required number to bring a list’s sum to zero.
- Write a Python program to implement a function that returns the negation of the total sum of a list.
Go to:
Previous: Find numbers that are adjacent to a prime number in the list, sorted without duplicates.
Next: Find the dictionary key whose case is different than all other keys.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.