Python: A valid filename
Validate Filenames
A valid filename should end in .txt, .exe, .jpg, .png, or .dll, and should have at most three digits, no additional periods. Write a Python program to create a list of True/False that determine whether candidate filename is valid or not.
Input: ['abc.txt', 'windows.dll', 'tiger.png', 'rose.jpg', 'test.py', 'win32.exe'] Output: ['Yes', 'Yes', 'Yes', 'Yes', 'No', 'Yes'] Input: ['.txt', 'windows.exe', 'tiger.jpeg', 'rose.c', 'test.java'] Output: ['No', 'Yes', 'No', 'No', 'No']
Visual Presentation:
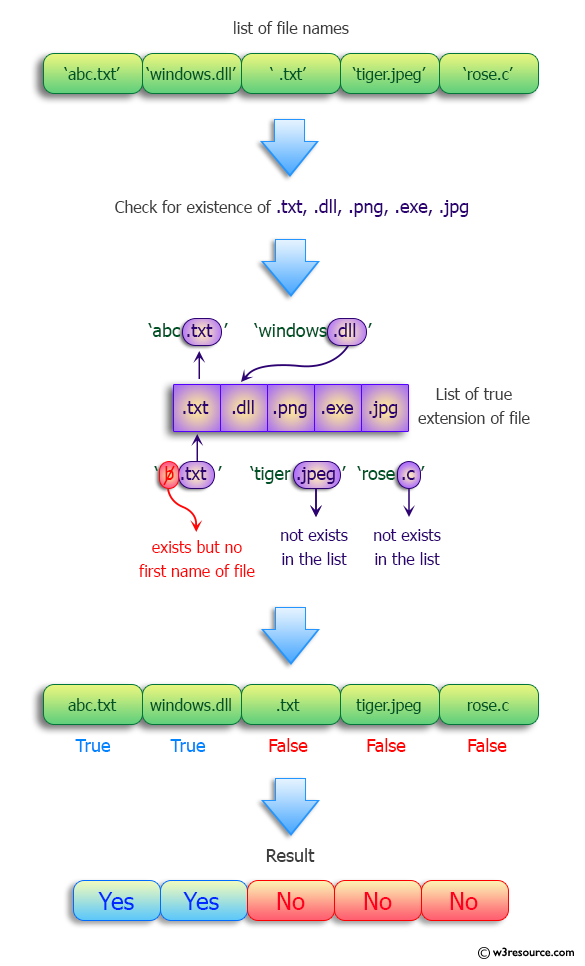
Sample Solution-1:
Python Code:
# Define a function named 'test' that takes a list of file names as input
def test(file_names):
# Use a list comprehension to iterate over each file name in the input list
return ["Yes" if
# Check conditions for a valid file name:
# - The file extension is one of ['txt', 'png', 'dll', 'exe', 'jpg']
# - The first character of the file name is alphabetic
# - The number of digits in the file name is less than 4
f.split(".")[1:] in [['txt'], ['png'], ['dll'], ['exe'], ['jpg']] and f[0].isalpha() and sum(c.isdigit() for c in f) < 4
# Return "Yes" if all conditions are met, otherwise "No"
else "No"
# Iterate over each file name 'f' in the input list
for f in file_names]
# Set a list of file names
file_names = ['abc.txt', 'windows.dll', 'tiger.png', 'rose.jpg', 'test.py', 'win32.exe']
# Print a message indicating the original list of files
print("Original list of files:")
# Print the original list of file names
print(file_names)
# Print a message indicating valid filenames and use the 'test' function to determine validity
print("Valid filenames:")
# Print the result of the 'test' function applied to the file names
print(test(file_names))
# Set another list of file names
file_names = ['.txt', 'windows.exe', 'tiger.jpeg', 'rose.c', 'test.java']
# Print a message indicating the original list of files
print("\nOriginal list of files:")
# Print the original list of file names
print(file_names)
# Print a message indicating valid filenames and use the 'test' function to determine validity
print("Valid filenames:")
# Print the result of the 'test' function applied to the file names
print(test(file_names))
Sample Output:
Original list of files: ['abc.txt', 'windows.dll', 'tiger.png', 'rose.jpg', 'test.py', 'win32.exe'] Valid filenames: ['Yes', 'Yes', 'Yes', 'Yes', 'No', 'Yes'] Original list of files: ['.txt', 'windows.exe', 'tiger.jpeg', 'rose.c', 'test.java'] Valid filenames: ['No', 'Yes', 'No', 'No', 'No']
Flowchart:
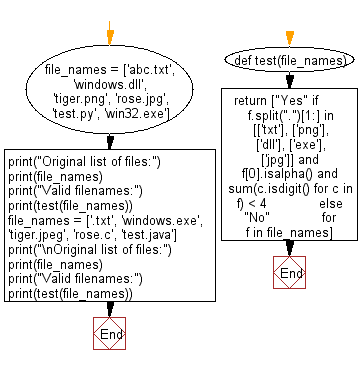
Sample Solution-2:
Python Code:
# Define a function named 'test' that takes a list of file names as input
def test(file_names):
# Initialize an empty list to store the validity status of each file name
valids = []
# Iterate over each file name in the input list using a for loop
for sat in file_names:
# Count the number of digits in the current file name
n_digits = sum(c.isdigit() for c in sat)
# Check conditions for a valid file name:
# - The file extension is one of ['txt', 'dll', 'exe']
# - The first character of the file name is alphabetic
# - The number of digits in the file name is less than or equal to 3
if sat.split(".")[1:] not in [['txt'], ['dll'], ['exe']] or not sat[0].isalpha() or n_digits > 3:
# Append "No" to the 'valids' list if the conditions are not met
valids.append("No")
else:
# Append "Yes" to the 'valids' list if all conditions are met
valids.append("Yes")
# Return the list of validity statuses
return valids
# Set a list of file names
file_names = ['abc.txt', 'windows.dll', 'tiger.png', 'rose.jpg', 'test.py', 'win32.exe']
# Print a message indicating the original list of files
print("Original list of files:")
# Print the original list of file names
print(file_names)
# Print a message indicating valid filenames and use the 'test' function to determine validity
print("Valid filenames:")
# Print the result of the 'test' function applied to the file names
print(test(file_names))
# Set another list of file names
file_names = ['.txt', 'windows.exe', 'tiger.jpeg', 'rose.c', 'test.java']
# Print a message indicating the original list of files
print("\nOriginal list of files:")
# Print the original list of file names
print(file_names)
# Print a message indicating valid filenames and use the 'test' function to determine validity
print("Valid filenames:")
# Print the result of the 'test' function applied to the file names
print(test(file_names))
Sample Output:
Original list of files: ['abc.txt', 'windows.dll', 'tiger.png', 'rose.jpg', 'test.py', 'win32.exe'] Valid filenames: ['Yes', 'Yes', 'No', 'No', 'No', 'Yes'] Original list of files: ['.txt', 'windows.exe', 'tiger.jpeg', 'rose.c', 'test.java'] Valid filenames: ['No', 'Yes', 'No', 'No', 'No']
Flowchart:
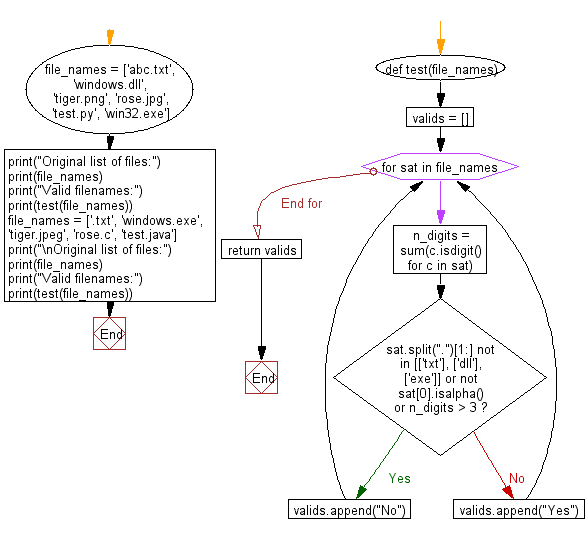
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Biggest even number between two numbers inclusive.
Next: Find numbers that are adjacent to a prime number in the list, sorted without duplicates.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics