Python: Find the first n Fibonacci numbers
Python Programming Puzzles: Exercise-51 with Solution
Write a Python program to find the product of the units digits in the numbers in a given list.
Input: 10 Output: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55] Input: 15 Output: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610] Input: 50 Output: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, 196418, 317811, 514229, 832040, 1346269, 2178309, 3524578, 5702887, 9227465, 14930352, 24157817, 39088169, 63245986, 102334155, 165580141, 267914296, 433494437, 701408733, 1134903170, 1836311903, 2971215073, 4807526976, 7778742049, 12586269025]
Sample Solution-1:
Python Code:
# Define a function named 'test' that generates Fibonacci numbers
def test(n):
# Initialize a list 'a' with the first two Fibonacci numbers
a = [1, 1]
# Continue adding Fibonacci numbers to the list until it reaches the desired count 'n'
while len(a) < n:
# Append the sum of the last two numbers in the list to generate the next Fibonacci number
a += [sum(a[-2:])]
# Return the first 'n' Fibonacci numbers
return a[:n]
# Set the value of 'n' to 10
n = 10
# Print a message indicating the task and the value of 'n'
print("\nFind the first",n,"Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
# Set the value of 'n' to 15
n = 15
# Print a message indicating the task and the value of 'n'
print("\nFind the first",n,"Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
# Set the value of 'n' to 50
n = 50
# Print a message indicating the task and the value of 'n'
print("\nFind the first",n,"Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
Sample Output:
Find the first 10 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55] Find the first 15 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610] Find the first 50 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, 196418, 317811, 514229, 832040, 1346269, 2178309, 3524578, 5702887, 9227465, 14930352, 24157817, 39088169, 63245986, 102334155, 165580141, 267914296, 433494437, 701408733, 1134903170, 1836311903, 2971215073, 4807526976, 7778742049, 12586269025]
Flowchart:
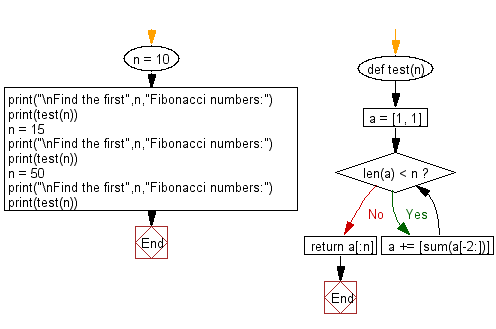
Sample Solution-2:
Python Code:
# Define a function named 'test' that generates Fibonacci numbers
def test(n):
# Initialize a list 'result' with the first two Fibonacci numbers
result = [1, 1]
# Continue adding Fibonacci numbers to the list until it reaches the desired count 'n'
while len(result) < n:
# Append the sum of the last two numbers in the list to generate the next Fibonacci number
result.append(result[-1] + result[-2])
# Return the first 'n' Fibonacci numbers
return result
# Set the value of 'n' to 10
n = 10
# Print a message indicating the task and the value of 'n'
print("Find the first", n, "Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
# Set the value of 'n' to 15
n = 15
# Print a message indicating the task and the value of 'n'
print("\nFind the first", n, "Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
# Set the value of 'n' to 50
n = 50
# Print a message indicating the task and the value of 'n'
print("\nFind the first", n, "Fibonacci numbers:")
# Print the result of the test function applied to 'n'
print(test(n))
Sample Output:
Find the first 10 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55] Find the first 15 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610] Find the first 50 Fibonacci numbers: [1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, 610, 987, 1597, 2584, 4181, 6765, 10946, 17711, 28657, 46368, 75025, 121393, 196418, 317811, 514229, 832040, 1346269, 2178309, 3524578, 5702887, 9227465, 14930352, 24157817, 39088169, 63245986, 102334155, 165580141, 267914296, 433494437, 701408733, 1134903170, 1836311903, 2971215073, 4807526976, 7778742049, 12586269025]
Flowchart:
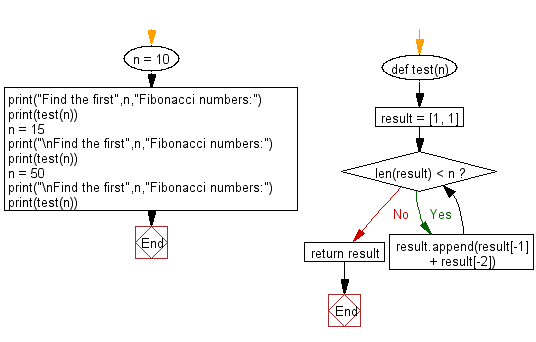
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the even-length words and sort them by length.
Next: Reverse the case of all strings. For those strings, which contain no letters, reverse the strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics