Python: Find the h-index, the largest positive number h such that h occurs in the sequence at least h times
H-Index Finder
Write a Python program to find the h-index, the largest positive number h such that h occurs in the sequence at least h times. If there is no such positive number return h = -1.
Input: [1, 2, 2, 3, 3, 4, 4, 4, 4] Output: 4 Input: [1, 2, 2, 3, 4, 5, 6] Output: 2 Input: [3, 1, 4, 17, 5, 17, 2, 1, 41, 32, 2, 5, 5, 5, 5] Output: 5
Visual Presentation:
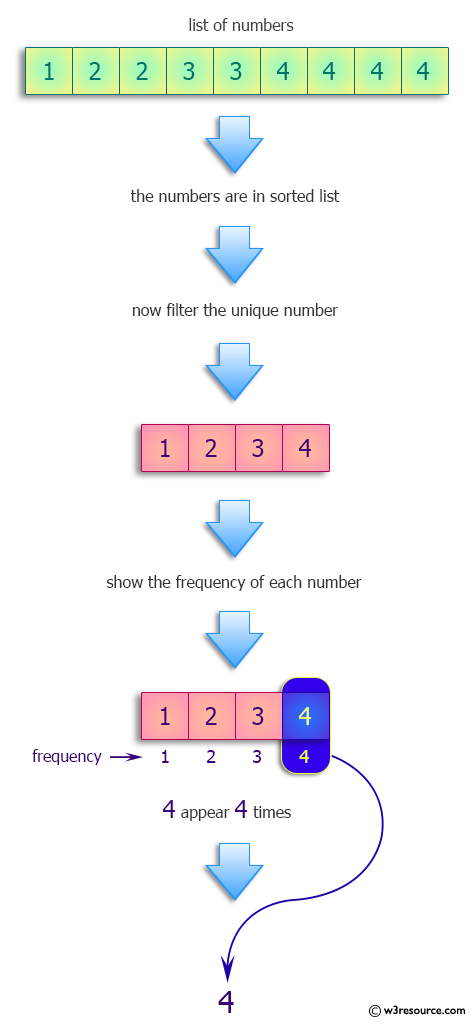
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Calculate the h-index, which is the largest positive number h
# such that h occurs in the sequence at least h times
return max([-1] + [i for i in nums if i > 0 and nums.count(i) >= i])
# Assign a specific list of numbers to the variable 'nums'
nums = [1, 2, 2, 3, 3, 4, 4, 4, 4]
# Print a message indicating the original list of numbers
print("Original list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the h-index calculation
print("h-index, the largest positive number h such that h occurs in the said sequence at least h times:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1,2,2,3,4,5,6]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the h-index calculation
print("h-index, the largest positive number h such that h occurs in the said sequence at least h times:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [3, 1, 4, 17, 5, 17, 2, 1, 41, 32, 2, 5, 5, 5, 5]
# Print a message indicating the original list of numbers
print("\nOriginal list of numbers:")
# Print the original list of numbers
print(nums)
# Print a message indicating the h-index calculation
print("h-index, the largest positive number h such that h occurs in the said sequence at least h times:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list of numbers: [1, 2, 2, 3, 3, 4, 4, 4, 4] h-index, the largest positive number h such that h occurs in the said sequence at least h times: 4 Original list of numbers: [1, 2, 2, 3, 4, 5, 6] h-index, the largest positive number h such that h occurs in the said sequence at least h times: 2 Original list of numbers: [3, 1, 4, 17, 5, 17, 2, 1, 41, 32, 2, 5, 5, 5, 5] h-index, the largest positive number h such that h occurs in the said sequence at least h times: 5
Flowchart:
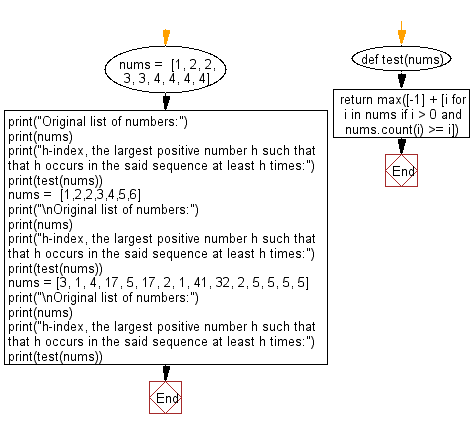
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the indices of two entries that show that the list is not in increasing order.
Next: Find the even-length words and sort them by length.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics