Python: Filter for the numbers in a list whose sum of digits is >0, where the first digit can be negative
Filter by Sum of Digits
Write a Python program to filter for numbers in a given list whose sum of digits is > 0, where the first digit can be negative.
Input: [11, -6, -103, -200] Output: [11, -103] Input: [1, 7, -4, 4, -9, 2] Output: [1, 7, 4, 2] Input: [10, -11, -71, -13, 14, -32] Output: [10, -13, 14]
Sample Solution:
Python Code:
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Use a list comprehension to filter numbers based on the sum of their digits
return [n for n in nums if int(str(n)[:2]) + sum(map(int, str(n)[2:])) > 0]
# Assign a specific list of numbers to the variable 'nums'
nums = [11, -6, -103, -200]
# Print a message indicating the original list of numbers
print("Original list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the numbers whose sum of digits is >0, allowing negative first digits
print("Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [1, 7, -4, 4, -9, 2]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the numbers whose sum of digits is >0, allowing negative first digits
print("Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of numbers to the variable 'nums'
nums = [10, -11, -71, -13, 14, -32]
# Print a message indicating the original list of numbers
print("\nOriginal list:")
# Print the original list of numbers
print(nums)
# Print a message indicating the numbers whose sum of digits is >0, allowing negative first digits
print("Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list: [11, -6, -103, -200] Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative: [11, -103] Original list: [1, 7, -4, 4, -9, 2] Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative: [1, 7, 4, 2] Original list: [10, -11, -71, -13, 14, -32] Find the numbers in the said list whose sum of digits is >0, where the first digit can be negative: [10, -13, 14]
Flowchart:
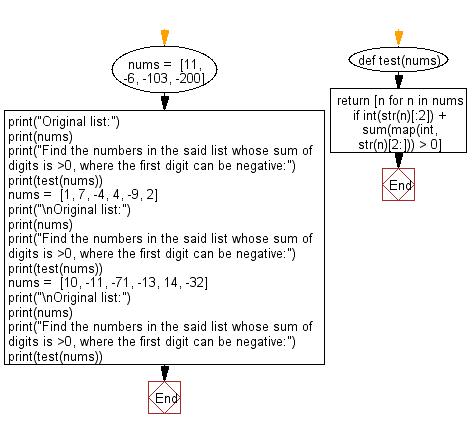
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the minimum even value and its index from a given array of numbers.
Next: Find the indices of two entries that show that the list is not in increasing order.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics