Python: Determine which characters of a hexadecimal number correspond to prime numbers
Hexadecimal Prime Characters
From Wikipedia:
The hexadecimal numeral system, often shortened to "hex", is a numeral system made up of 16 symbols (base 16). The standard numeral system is called decimal (base 10) and uses ten symbols: 0,1,2,3,4,5,6,7,8,9. Hexadecimal uses the decimal numbers and six extra symbols. There are no numerical symbols that represent values greater than nine, so letters taken from the English alphabet are used, specifically A, B, C, D, E and F. Hexadecimal A = decimal 10, and hexadecimal F = decimal 15.
A prime number (or a prime) is a natural number greater than 1 that is not a product of two smaller natural numbers. A natural number greater than 1 that is not prime is called a composite number. For example, 5 is prime because the only ways of writing it as a product, 1 × 5 or 5 × 1, involve 5 itself. However, 4 is composite because it is a product (2 × 2) in which both numbers are smaller than 4. Primes are central in number theory because of the fundamental theorem of arithmetic: every natural number greater than 1 is either a prime itself or can be factorized as a product of primes that is unique up to their order.
Write a Python program to find which characters of a hexadecimal number correspond to prime numbers.
Input: 123ABCD Output: [False, True, True, False, True, False, True] Input: 123456 Output: [False, True, True, False, True, False] Input: FACE Output: [False, False, False, False]
Visual Presentation:
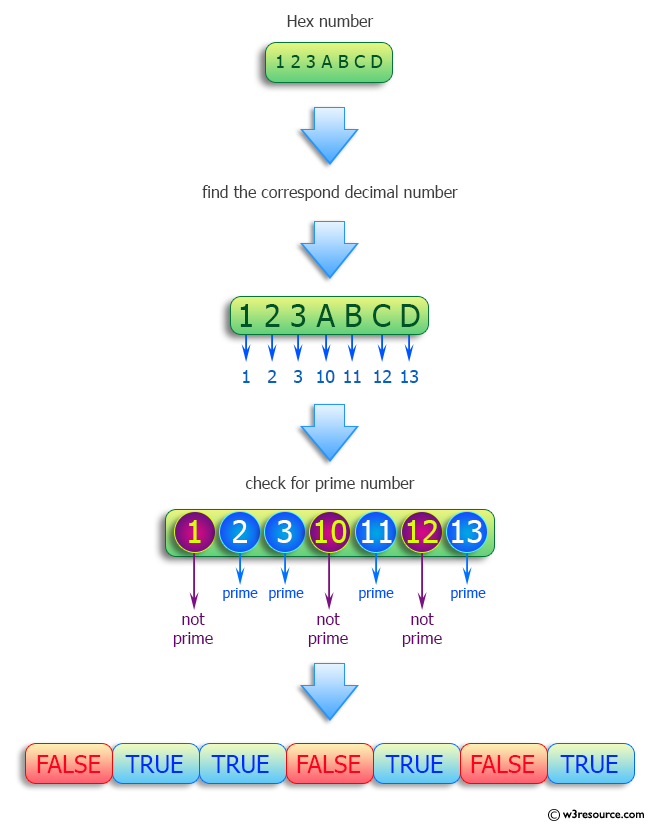
Sample Solution:
Python Code:
# Define a function named 'test' that takes a hexadecimal number 'hn' as input
def test(hn):
# List comprehension to check if each character in 'hn' corresponds to a prime number or 'B' or 'D'
return [c in "2357BD" for c in hn]
# Assign a specific hexadecimal number 'hn' to the variable
hn = "123ABCD"
# Print a message indicating the original hexadecimal number
print("Original hexadecimal number:", hn)
# Print a message indicating the operation to be performed
print("Characters of the said hexadecimal number correspond to prime numbers:")
# Print the result of the test function applied to 'hn'
print(test(hn))
# Assign another specific hexadecimal number 'hn' to the variable
hn = "123456"
# Print a message indicating the original hexadecimal number
print("\nOriginal hexadecimal number:", hn)
# Print a message indicating the operation to be performed
print("Characters of the said hexadecimal number correspond to prime numbers:")
# Print the result of the test function applied to 'hn'
print(test(hn))
# Assign another specific hexadecimal number 'hn' to the variable
hn = "FACE"
# Print a message indicating the original hexadecimal number
print("\nOriginal hexadecimal number:", hn)
# Print a message indicating the operation to be performed
print("Characters of the said hexadecimal number correspond to prime numbers:")
# Print the result of the test function applied to 'hn'
print(test(hn))
Sample Output:
Original hexadecimal number: 123ABCD Characters of the said hexadecimal number correspond to prime numbers: [False, True, True, False, True, False, True] Original hexadecimal number: 123456 Characters of the said hexadecimal number correspond to prime numbers: [False, True, True, False, True, False] Original hexadecimal number: FACE Characters of the said hexadecimal number correspond to prime numbers: [False, False, False, False]
Flowchart:
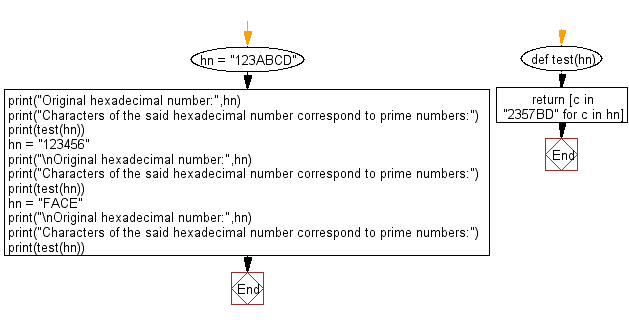
For more Practice: Solve these Related Problems:
- Write a Python program to convert a hexadecimal string into its digit values and check which correspond to prime numbers.
- Write a Python program to iterate over each character of a hexadecimal number and output True if its decimal equivalent is prime.
- Write a Python program to use a helper function to test prime status for each digit in a hexadecimal string.
- Write a Python program to map each hexadecimal digit to a boolean indicating whether its value is prime.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find all words in a given string with n consonants.
Next: Find all even palindromes up to n.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics