Python: Find string s that, when case is flipped gives target where vowels are replaced by chars two later
Flip Case and Shift Vowels
Write a Python program to find strings that, when case is flipped, give a target where vowels are replaced by characters two later.
Input: Python Output: pYTHQN Input: aeiou Output: CGKQW Input: Hello, world! Output: hGLLQ, WQRLD! Input: AEIOU Output: cgkqw
Visual Presentation:
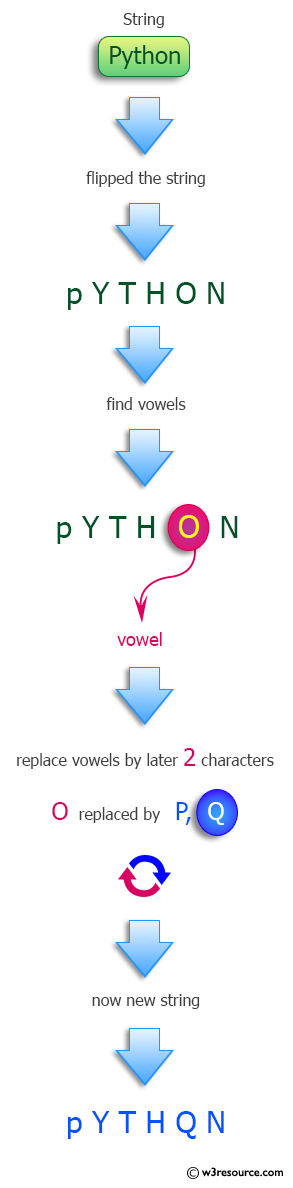
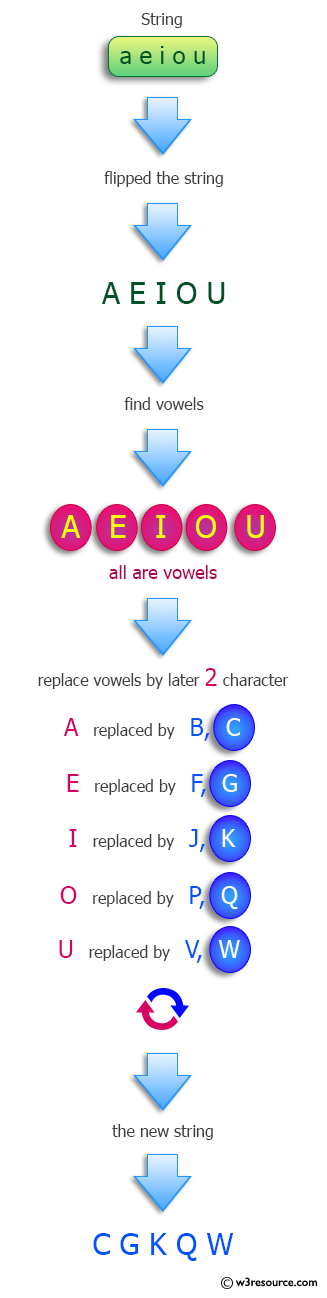
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Use the translate method to create a mapping for each vowel to the character two positions later
translation_mapping = {ord(c): ord(c) + 2 for c in "aeiouAEIOU"}
# Apply the translation mapping and swap the case of the characters in the string
result = strs.translate(translation_mapping).swapcase()
# Return the modified string
return result
# Assign a specific string 'strs' to the variable
strs = "Python"
# Print a message indicating the original string
print("Original string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "aeiou"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "Hello, world!"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "AEIOU"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
Sample Output:
Original string: Python Find string s that, when case is flipped gives target where vowels are replaced by chars two later: pYTHQN Original string: aeiou Find string s that, when case is flipped gives target where vowels are replaced by chars two later: CGKQW Original string: Hello, world! Find string s that, when case is flipped gives target where vowels are replaced by chars two later: hGLLQ, WQRLD! Original string: AEIOU Find string s that, when case is flipped gives target where vowels are replaced by chars two later: cgkqw
Flowchart:
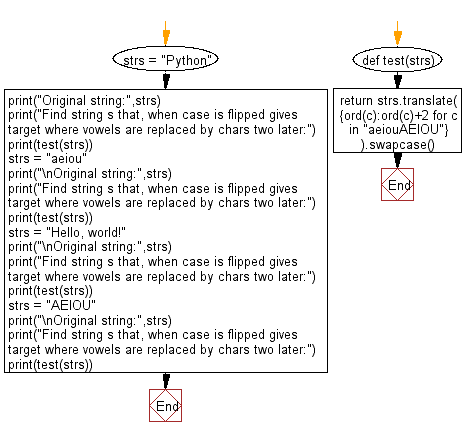
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a string 'strs' as input
def test(strs):
# Use the swapcase method to flip the case of characters and create a translation mapping
# Replace vowels with characters two positions later in the alphabet
translation_mapping = {
ord('a'): ord('c'),
ord('e'): ord('g'),
ord('i'): ord('j'),
ord('o'): ord('q'),
ord('u'): ord('x'),
ord('A'): ord('C'),
ord('E'): ord('G'),
ord('I'): ord('J'),
ord('O'): ord('Q'),
ord('U'): ord('X'),
ord(' '): ord(' ')
}
# Apply the swapcase and translation mappings to the string
result = strs.swapcase().translate(translation_mapping)
# Return the modified string
return result
# Assign a specific string 'strs' to the variable
strs = "Python"
# Print a message indicating the original string
print("Original string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "aeiou"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "Hello, world!"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
# Assign another specific string 'strs' to the variable
strs = "AEIOU"
# Print a message indicating the original string
print("\nOriginal string:", strs)
# Print a message indicating the operation to be performed
print("Find string s that, when case is flipped gives target where vowels are replaced by chars two later:")
# Print the result of the test function applied to 'strs'
print(test(strs))
Sample Output:
Original string: Python Find string s that, when case is flipped gives target where vowels are replaced by chars two later: pYTHQN Original string: aeiou Find string s that, when case is flipped gives target where vowels are replaced by chars two later: CGJQX Original string: Hello, world! Find string s that, when case is flipped gives target where vowels are replaced by chars two later: hGLLQ, WQRLD! Original string: AEIOU Find string s that, when case is flipped gives target where vowels are replaced by chars two later: cgjqx
Flowchart:
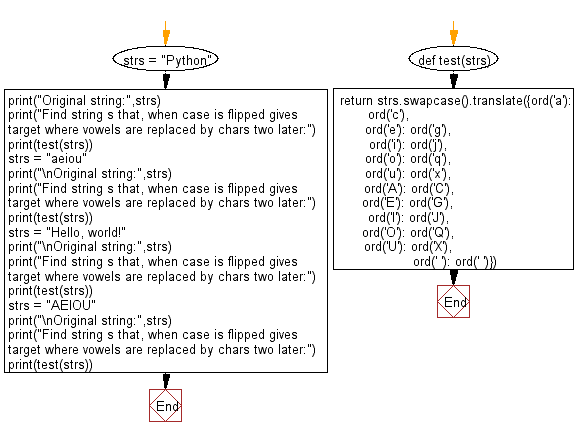
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Determine which triples sum to zero.
Next: Sort numbers based on strings.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics