Python: Determine which triples sum to zero
Zero-Sum Triples Finder
Write a Python program to determine which triples sum to zero from a given list of lists.
Input: [[1343532, -2920635, 332], [-27, 18, 9], [4, 0, -4], [2, 2, 2], [-20, 16, 4]] Output: [False, True, True, False, True] Input: [[1, 2, -3], [-4, 0, 4], [0, 1, -5], [1, 1, 1], [-2, 4, -1]] Output: [True, True, False, False, False]
Visual Presentation:
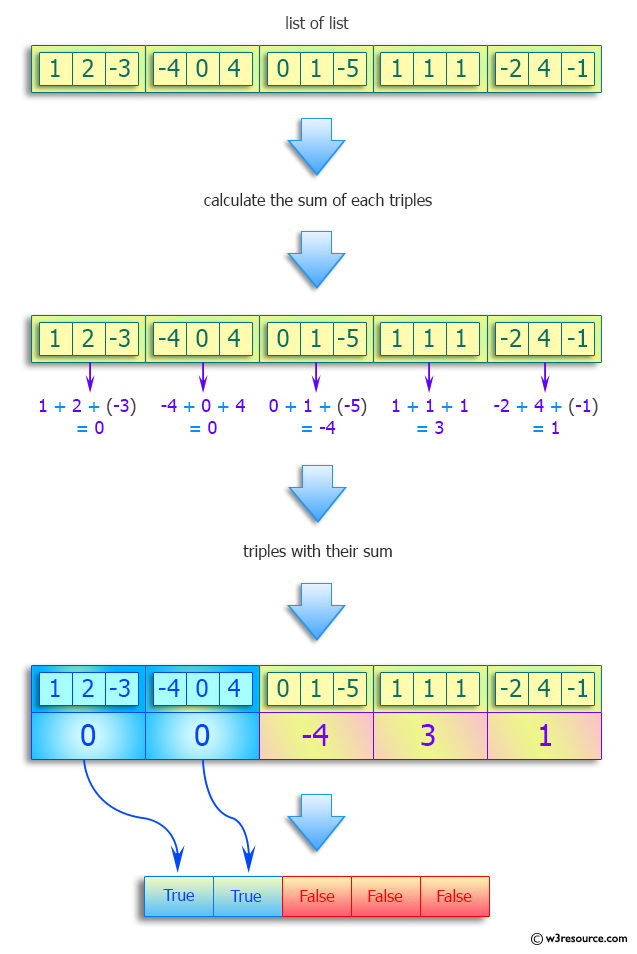
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of lists of numbers 'nums' as input
def test(nums):
# Use a list comprehension to check if the sum of each triple in 'nums' is equal to zero
return [sum(t) == 0 for t in nums]
# Assign a specific list of lists 'nums' to the variable
nums = [[1343532, -2920635, 332], [-27, 18, 9], [4, 0, -4], [2, 2, 2], [-20, 16, 4]]
# Print a message indicating the original list of lists
print("Original list of lists:", nums)
# Print a message indicating the operation to be performed
print("Determine which triples sum to zero:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of lists 'nums' to the variable
nums = [[1, 2, -3], [-4, 0, 4], [0, 1, -5], [1, 1, 1], [-2, 4, -1]]
# Print a message indicating the original list of lists
print("\nOriginal list of lists:", nums)
# Print a message indicating the operation to be performed
print("Determine which triples sum to zero:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list of lists: [[1343532, -2920635, 332], [-27, 18, 9], [4, 0, -4], [2, 2, 2], [-20, 16, 4]] Determine which triples sum to zero: [False, True, True, False, True] Original list of lists: [[1, 2, -3], [-4, 0, 4], [0, 1, -5], [1, 1, 1], [-2, 4, -1]] Determine which triples sum to zero: [True, True, False, False, False]
Flowchart:
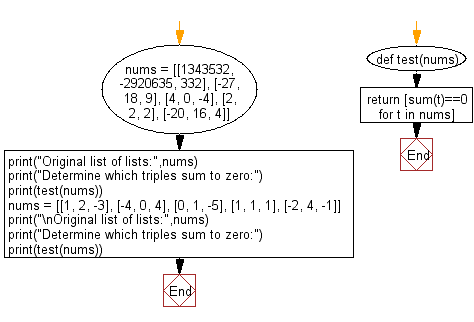
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of lists of numbers 'nums' as input
def test(nums):
# Initialize an empty list to store Boolean values indicating if a triple sums to zero
zero_sums = []
# Iterate through each triple in the list of lists 'nums'
for trip in nums:
# Iterate through each pair of indices i, j in the triple
for i in range(len(trip)):
for j in range(i + 1, len(trip)):
# Iterate through each index k greater than j
for k in range(j + 1, len(trip)):
# Check if the sum of elements at indices i, j, and k is equal to zero
if trip[i] + trip[j] + trip[k] == 0:
# If a zero sum is found, append True to the 'zero_sums' list and break the loop
zero_sums.append(True)
break
else:
continue
break
else:
continue
break
else:
# If no zero sum is found, append False to the 'zero_sums' list
zero_sums.append(False)
# Return the list of Boolean values indicating whether each triple sums to zero
return zero_sums
# Assign a specific list of lists 'nums' to the variable
nums = [[1343532, -2920635, 332], [-27, 18, 9], [4, 0, -4], [2, 2, 2], [-20, 16, 4]]
# Print a message indicating the original list of lists
print("Original list of lists:", nums)
# Print a message indicating the operation to be performed
print("Determine which triples sum to zero:")
# Print the result of the test function applied to 'nums'
print(test(nums))
# Assign another specific list of lists 'nums' to the variable
nums = [[1, 2, -3], [-4, 0, 4], [0, 1, -5], [1, 1, 1], [-2, 4, -1]]
# Print a message indicating the original list of lists
print("\nOriginal list of lists:", nums)
# Print a message indicating the operation to be performed
print("Determine which triples sum to zero:")
# Print the result of the test function applied to 'nums'
print(test(nums))
Sample Output:
Original list of lists: [[1343532, -2920635, 332], [-27, 18, 9], [4, 0, -4], [2, 2, 2], [-20, 16, 4]] Determine which triples sum to zero: [False, True, True, False, True] Original list of lists: [[1, 2, -3], [-4, 0, 4], [0, 1, -5], [1, 1, 1], [-2, 4, -1]] Determine which triples sum to zero: [True, True, False, False, False]
Flowchart:
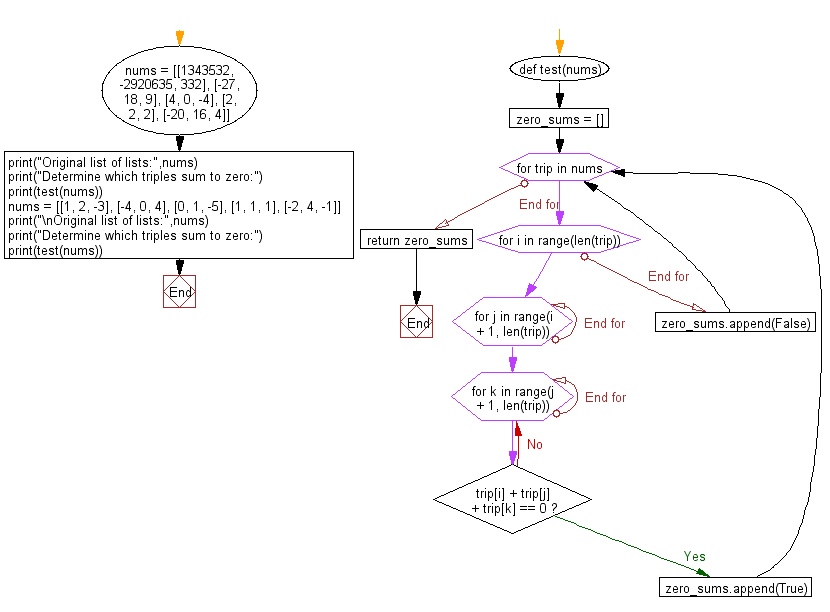
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Sort the numbers by the sum of their digits.
Next: Find string s that, when case is flipped gives target where vowels are replaced by chars two later.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics