Python: Find the largest k numbers
Find Largest K Numbers
Write a Python program to find the largest k numbers from a given list of numbers.
Input: [1, 2, 3, 4, 5, 5, 3, 6, 2] Output: [6] Input: [1, 2, 3, 4, 5, 5, 3, 6, 2] Output: [6, 5] Input: [1, 2, 3, 4, 5, 5, 3, 6, 2] Output: [6, 5, 5] Input: [1, 2, 3, 4, 5, 5, 3, 6, 2] Output: [6, 5, 5, 4] Input: [1, 2, 3, 4, 5, 5, 3, 6, 2] Output: [6, 5, 5, 4, 3]
Visual Presentation:
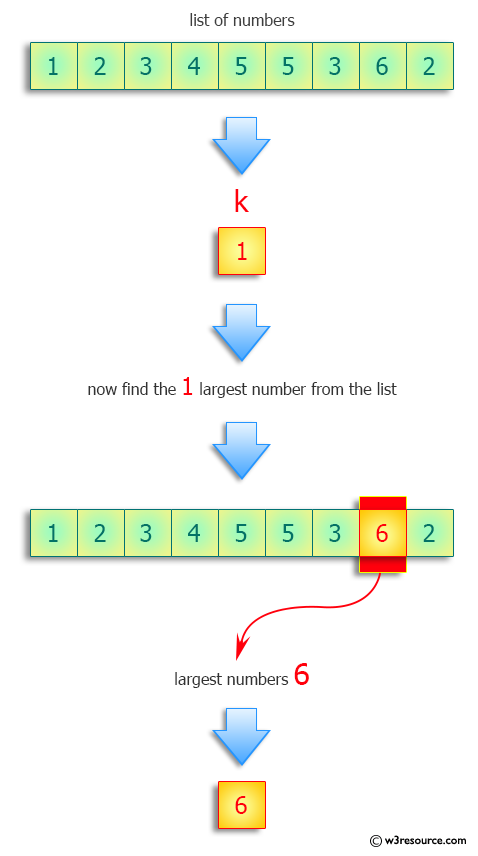
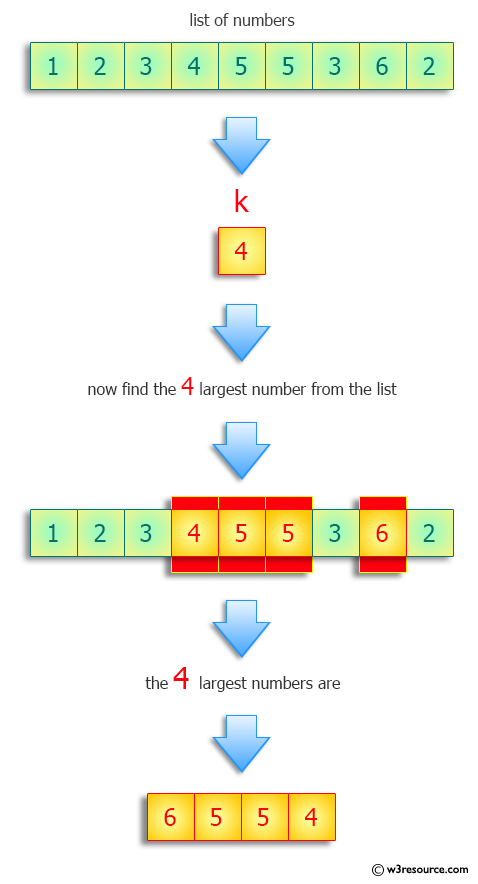
Sample Solution-1:
Python Code:
#License: https://bit.ly/3oLErEI
def test(nums, k):
if k == 0:
return []
elif k == len(nums):
return nums
else:
x = nums[0]
for n in nums:
if x < n:
x = n
result = [x]
largest = nums[:]
largest.remove(x)
while len(result) != k:
smallest = largest[0]
for n in largest:
if smallest < n:
smallest = n
result.append(smallest)
largest.remove(smallest)
return result
nums = [1, 2, 3, 4, 5, 5, 3, 6, 2]
print("Original list of numbers:",nums)
k = 1
print("Largest", k, "numbers from the said list:")
print(test(nums, k))
k = 2
print("Largest", k, "numbers from the said list:")
print(test(nums, k))
k = 3
print("Largest", k, "numbers from the said list:")
print(test(nums, k))
k = 4
print("Largest", k, "numbers from the said list:")
print(test(nums, k))
k = 5
print("Largest", k, "numbers from the said list:")
print(test(nums, k))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 5, 3, 6, 2] Largest 1 numbers from the said list: [6] Largest 2 numbers from the said list: [6, 5] Largest 3 numbers from the said list: [6, 5, 5] Largest 4 numbers from the said list: [6, 5, 5, 4] Largest 5 numbers from the said list: [6, 5, 5, 4, 3]
Flowchart:
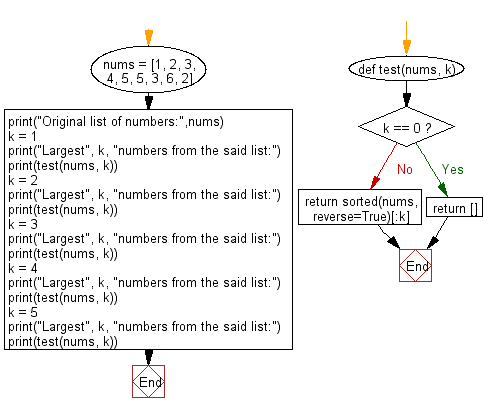
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' and an integer 'k' as input
def test(nums, k):
# Check if k is zero, in which case an empty list is returned
if k == 0:
return []
# Check if k is equal to the length of the list, in which case the original list is returned
elif k == len(nums):
return nums
else:
# Initialize variable 'x' to the first element of the list
x = nums[0]
# Find the maximum element in the list and assign it to 'x'
for n in nums:
if x < n:
x = n
# Initialize a list 'result' with the maximum element as its first element
result = [x]
# Create a copy of the original list and assign it to 'largest'
largest = nums[:]
# Remove the maximum element from 'largest'
largest.remove(x)
# Continue adding elements to 'result' until its length becomes equal to 'k'
while len(result) != k:
# Find the smallest element in 'largest' and assign it to 'smallest'
smallest = largest[0]
for n in largest:
if smallest < n:
smallest = n
# Add the smallest element to 'result' and remove it from 'largest'
result.append(smallest)
largest.remove(smallest)
return result
# Assign a specific list of numbers 'nums' to the variable
nums = [1, 2, 3, 4, 5, 5, 3, 6, 2]
# Print a message indicating the original list of numbers
print("Original list of numbers:", nums)
# Assign a specific value 'k' to the variable
k = 1
# Print a message indicating the operation to be performed
print("Largest", k, "numbers from the said list:")
# Print the result of the test function applied to 'nums' and 'k'
print(test(nums, k))
# Assign another specific value 'k' to the variable
k = 2
# Print a message indicating the operation to be performed
print("Largest", k, "numbers from the said list:")
# Print the result of the test function applied to 'nums' and 'k'
print(test(nums, k))
# Assign another specific value 'k' to the variable
k = 3
# Print a message indicating the operation to be performed
print("Largest", k, "numbers from the said list:")
# Print the result of the test function applied to 'nums' and 'k'
print(test(nums, k))
# Assign another specific value 'k' to the variable
k = 4
# Print a message indicating the operation to be performed
print("Largest", k, "numbers from the said list:")
# Print the result of the test function applied to 'nums' and 'k'
print(test(nums, k))
# Assign another specific value 'k' to the variable
k = 5
# Print a message indicating the operation to be performed
print("Largest", k, "numbers from the said list:")
# Print the result of the test function applied to 'nums' and 'k'
print(test(nums, k))
Sample Output:
Original list of numbers: [1, 2, 3, 4, 5, 5, 3, 6, 2] Largest 1 numbers from the said list: [6] Largest 2 numbers from the said list: [6, 5] Largest 3 numbers from the said list: [6, 5, 5] Largest 4 numbers from the said list: [6, 5, 5, 4] Largest 5 numbers from the said list: [6, 5, 5, 4, 3]
Flowchart:
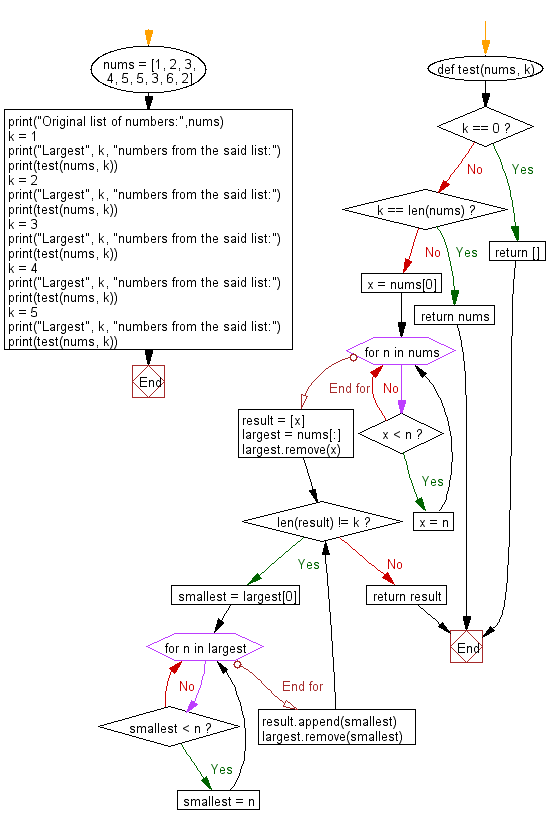
For more Practice: Solve these Related Problems:
- Write a Python program to return the largest k numbers from a list using sorting and slicing.
- Write a Python program to use a heap data structure to efficiently extract the k largest elements from a list.
- Write a Python program to implement a selection algorithm that finds the top k numbers without fully sorting the list.
- Write a Python program to use the nlargest() function from heapq to extract the largest k numbers from a list.
Go to:
Previous: Product of the odd digits in n, or 0 if there aren't any.
Next: Find the largest integer divisor of a number n that is less than n.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.