Python: Find the indices of two numbers that sum to 0 in a given list
Indices of Numbers Summing to Zero
Write a Python program to find the indices of two numbers that sum to 0 in a given list of numbers.
Input: [1, -4, 6, 7, 4] Output: [4, 1] Input: [1232, -20352, 12547, 12440, 741, 341, 525, 20352, 91, 20] Output: [1, 7]
Visual Presentation:
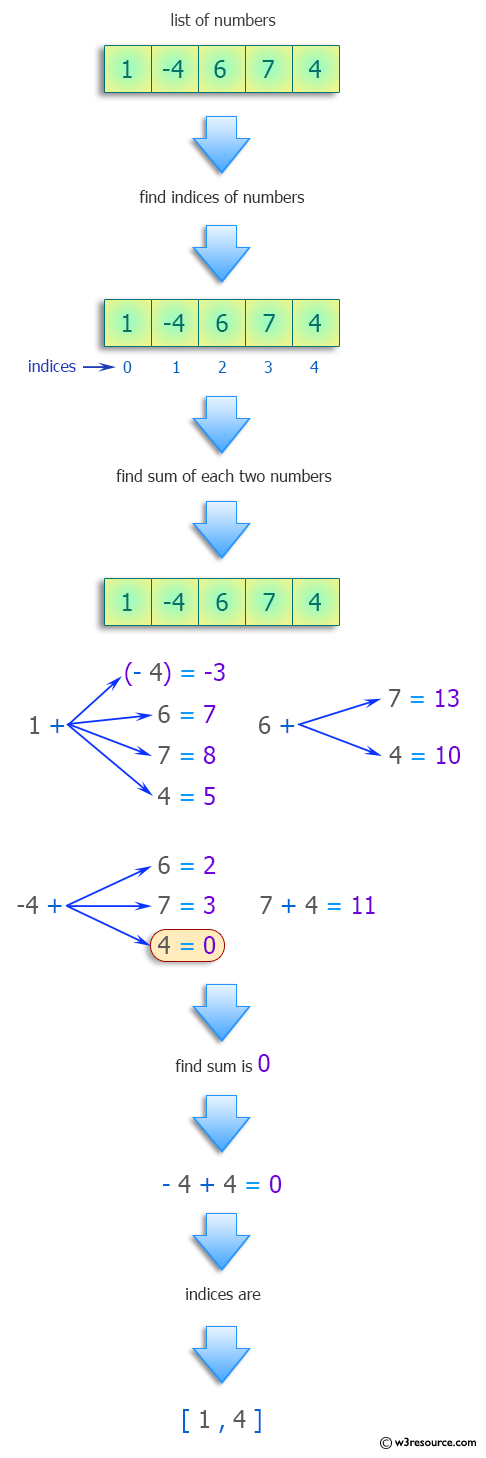
Sample Solution-1:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Create a set 's' from the given list 'nums'
s = set(nums)
# Iterate over each element 'i' in the set 's'
for i in s:
# Check if the negation of 'i' is also in the set 's'
if -i in s:
# If found, return the indices of 'i' and its negation in the original list 'nums'
return [nums.index(i), nums.index(-i)]
# Assign a specific list of numbers 'nums' to the variable
nums = [1, -4, 6, 7, 4]
# Print the original list of numbers 'nums'
print("Original List:")
print(nums)
# Print a message indicating the operation to be performed
print("Indices of two numbers that sum to 0 in the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign another specific list of numbers 'nums' to the variable
nums = [1232, -20352, 12547, 12440, 741, 341, 525, 20352, 91, 20]
# Print the original list of numbers 'nums'
print("\nOriginal List:")
print(nums)
# Print a message indicating the operation to be performed
print("Indices of two numbers that sum to 0 in the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
Sample Output:
Original List: [1, -4, 6, 7, 4] Indices of two numbers that sum to 0 in the said list: [4, 1] Original List: [1232, -20352, 12547, 12440, 741, 341, 525, 20352, 91, 20] Indices of two numbers that sum to 0 in the said list: [1, 7]
Flowchart:
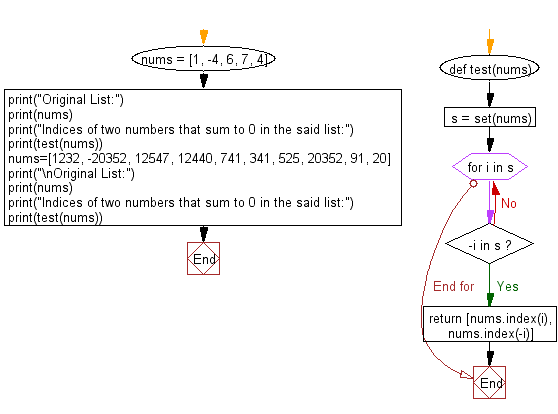
Sample Solution-2:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Initialize an empty list 'result' to store the indices
result = []
# Iterate over each index 'ind' in the range of the length of the list 'nums'
for ind in range(len(nums)):
# Iterate over each index 'i' in the range of the length of the list 'nums'
for i in range(len(nums)):
# Check if the current index 'ind' is not equal to the other index 'i'
# and if the sum of the numbers at the current index and the other index is zero
if ind != i and nums[ind] + nums[i] == 0:
# Append the current index 'ind' and the other index 'i' to the 'result' list
result.append(ind)
result.append(i)
# Found the indices; no need to go through the whole list
return result
# Assign a specific list of numbers 'nums' to the variable
nums = [1, -4, 6, 7, 4]
# Print the original list of numbers 'nums'
print("Original List:")
print(nums)
# Print a message indicating the operation to be performed
print("Indices of two numbers that sum to 0 in the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign another specific list of numbers 'nums' to the variable
nums = [1232, -20352, 12547, 12440, 741, 341, 525, 20352, 91, 20]
# Print the original list of numbers 'nums'
print("\nOriginal List:")
print(nums)
# Print a message indicating the operation to be performed
print("Indices of two numbers that sum to 0 in the said list:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
Sample Output:
Original List: [1, -4, 6, 7, 4] Indices of two numbers that sum to 0 in the said list: [1, 4] Original List: [1232, -20352, 12547, 12440, 741, 341, 525, 20352, 91, 20] Indices of two numbers that sum to 0 in the said list: [1, 7]
Flowchart:
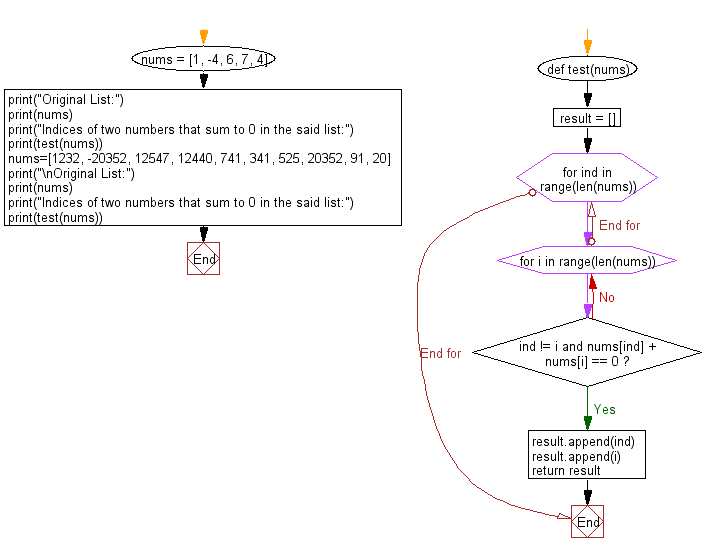
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Select a string from a given list of strings with the most unique characters.
Next: Find the list that has fewer total characters (including repetitions).
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics