Python: Find x that minimizes mean squared deviation from a given list of numbers
Minimize Mean Squared Deviation
Squared deviations from the mean (SDM) are involved in various calculations. In probability theory and statistics, the definition of variance is either the expected value of the SDM (when considering a theoretical distribution) or its average value (for actual experimental data). Computations for analysis of variance involve the partitioning of a sum of SDM.
Write a Python program to find x that minimizes the mean squared deviation from a given list of numbers.
The problem requires minimizing the sum of squared deviations, which turns out to be the mean mu. Moreover, if mu is the mean of the numbers then a simple calculation.
Input: [4, -5, 17, -9, 14, 108, -9] Output: 17.142857142857142 Input: [12, -2, 14, 3, -15, 10, -45, 3, 30] Output: 1.1111111111111112
Sample Solution:
Python Code:
# License: https://bit.ly/3oLErEI
# Define a function named 'test' that takes a list of numbers 'nums' as input
def test(nums):
# Calculate the mean by summing the numbers and dividing by the length of the list
return sum(nums) / len(nums)
# Assign a specific list of numbers 'nums' to the variable
nums = [4, -5, 17, -9, 14, 108, -9]
# Print the original list of numbers 'nums'
print("Original list:")
print(nums)
# Print a message indicating the operation to be performed
print("Minimizes mean squared deviation from the said list of numbers:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
# Assign another specific list of numbers 'nums' to the variable
nums = [12, -2, 14, 3, -15, 10, -45, 3, 30]
# Print the original list of numbers 'nums'
print("Original list:")
print(nums)
# Print a message indicating the operation to be performed
print("Minimizes mean squared deviation from the said list of numbers:")
# Print the result of the test function applied to the 'nums' list
print(test(nums))
Sample Output:
Original list: [4, -5, 17, -9, 14, 108, -9] Minimizes mean squared deviation from the said list of numbers: 17.142857142857142 Original list: [12, -2, 14, 3, -15, 10, -45, 3, 30] Minimizes mean squared deviation from the said list of numbers: 1.1111111111111112
Flowchart:
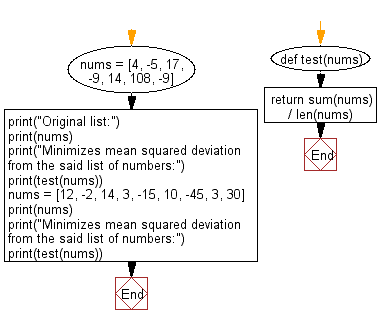
For more Practice: Solve these Related Problems:
- Write a Python program to find the value x that minimizes the mean squared deviation for a list of numbers by testing multiple candidates.
- Write a Python program to implement a brute-force search for the optimal x that minimizes the sum of squared differences.
- Write a Python program to use calculus concepts approximated with discrete values to minimize the mean squared deviation in a list.
- Write a Python program to iteratively adjust x to reduce the mean squared deviation of a given set of numbers.
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Find the largest number where commas or periods are decimal points.
Next: Select a string from a given list of strings with the most unique characters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics