Python: Split a string of words separated by commas and spaces into 2 lists: words and separators
Write a Python program to split a string of words separated by commas and spaces into two lists, words and separators.
Input: W3resource Python, Exercises.
Output:
[['W3resource', 'Python', '', 'Exercises.'], [' ', ',', ' ']]
Input: The dance, held in the school gym, ended at midnight.
Output:
[['The', 'dance', '', 'held', 'in', 'the', 'school', 'gym', '', 'ended', 'at', 'midnight.'], [' ', ',', ' ', ' ', ' ', ' ', ' ', ',', ' ', ' ', ' ']]
Sample Solution:
Python Code:
#License: https://bit.ly/3oLErEI
def test(s):
words = []
word = ""
for c in s:
if c in " ,":
words += [word, c]
word = ""
else:
word += c
words += [word]
return [words[::2], words[1::2]]
s = "W3resource Python, Exercises."
print("Original string:",s)
print("Split the said string into 2 lists: words and separators:")
print(test(s))
s = "The dance, held in the school gym, ended at midnight."
print("\nOriginal string:",s)
print("Split the said string into 2 lists: words and separators:")
print(test(s))
s = "The colors in my studyroom are blue, green, and yellow."
print("\nOriginal string:",s)
print("Split the said string into 2 lists: words and separators:")
print(test(s))
Sample Output:
Original string: W3resource Python, Exercises. Split the said string into 2 lists: words and separators: [['W3resource', 'Python', '', 'Exercises.'], [' ', ',', ' ']] Original string: The dance, held in the school gym, ended at midnight. Split the said string into 2 lists: words and separators: [['The', 'dance', '', 'held', 'in', 'the', 'school', 'gym', '', 'ended', 'at', 'midnight.'], [' ', ',', ' ', ' ', ' ', ' ', ' ', ',', ' ', ' ', ' ']] Original string: The colors in my studyroom are blue, green, and yellow. Split the said string into 2 lists: words and separators: [['The', 'colors', 'in', 'my', 'studyroom', 'are', 'blue', '', 'green', '', 'and', 'yellow.'], [' ', ' ', ' ', ' ', ' ', ' ', ',', ' ', ',', ' ', ' ']]
Flowchart:
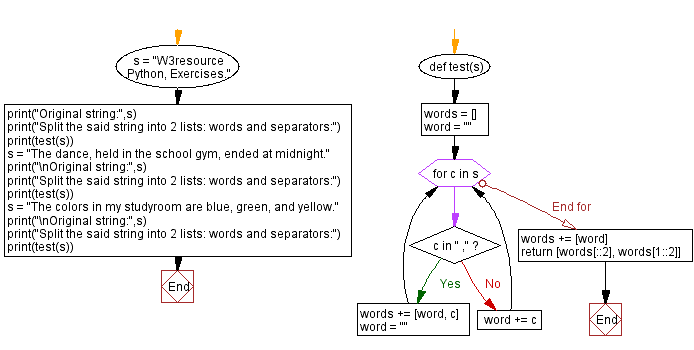
Python Code Editor :
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.