Pandas SQL Query: Display the name, and department number for all employees whose last name is "McEwen"
5. Employee Names & Dept No. for Last Name "McEwen"
Write a Pandas program to display the first and last name, and department number for all employees whose last name is "McEwen".
EMPLOYEES.csv
DEPARTMENTS.csv
Sample Solution :
Python Code :
import pandas as pd
employees = pd.read_csv(r"EMPLOYEES.csv")
departments = pd.read_csv(r"DEPARTMENTS.csv")
job_history = pd.read_csv(r"JOB_HISTORY.csv")
jobs = pd.read_csv(r"JOBS.csv")
countries = pd.read_csv(r"COUNTRIES.csv")
regions = pd.read_csv(r"REGIONS.csv")
locations = pd.read_csv(r"LOCATIONS.csv")
print("Last name First name Department ID")
result = employees[employees.last_name == 'McEwen']
for index, row in result.iterrows():
print(row['last_name'],' ',row['first_name'],' ',row['department_id'])
Sample Output:
Last name First name Department ID McEwen Allan 80.0
Equivalent SQL Syntax:
SELECT first_name, last_name, department_id FROM employees WHERE last_name = 'McEwen';
Click to view the table contain:
For more Practice: Solve these Related Problems:
- Write a Pandas program to display the first and last name, and department number for employees whose last name matches "McEwen" exactly (case sensitive).
- Write a Pandas program to display employee names and department number for employees with last name "McEwen", using a case-insensitive match.
- Write a Pandas program to display employee names and department number for "McEwen" after filtering out employees with null department numbers.
- Write a Pandas program to display employee names, department number, and salary for employees whose last name is "McEwen".
Go to:
Previous: Write a Pandas program to select distinct department id from employees file.
Next: Write a Pandas program to display the first, last name, salary and department number for those employees whose first name starts with the letter ‘S’.
Python Code Editor:
Structure of HR database :
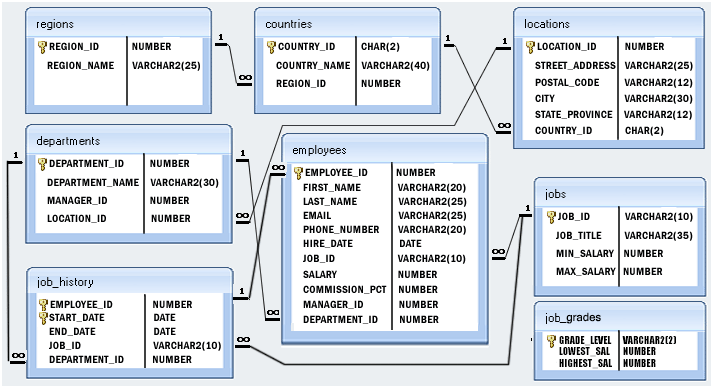
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?