Pandas SQL Query: Display the details of jobs in descending sequence on job title
Write a Pandas program to display the details of jobs in descending sequence on job title.
JOBS.csv
Sample Solution :
Python Code :
import pandas as pd
employees = pd.read_csv(r"EMPLOYEES.csv")
departments = pd.read_csv(r"DEPARTMENTS.csv")
job_history = pd.read_csv(r"JOB_HISTORY.csv")
jobs = pd.read_csv(r"JOBS.csv")
countries = pd.read_csv(r"COUNTRIES.csv")
regions = pd.read_csv(r"REGIONS.csv")
locations = pd.read_csv(r"LOCATIONS.csv")
print("job_id Job ID min_salary max_salary")
result = jobs.sort_values('job_title')
for index, row in result.iterrows():
print(row['job_id'].ljust(15),row['job_title'].ljust(35),str(row['min_salary']).ljust(9),row['max_salary'])
Sample Output:
job_id Job ID min_salary max_salary FI_ACCOUNT Accountant 4200 9000 AC_MGR Accounting Manager 8200 16000 AD_ASST Administration Assistant 3000 6000 AD_VP Administration Vice President 15000 30000 FI_MGR Finance Manager 8200 16000 HR_REP Human Resources Representative 4000 9000 MK_MAN Marketing Manager 9000 15000 MK_REP Marketing Representative 4000 9000 AD_PRES President 20000 40000 IT_PROG Programmer 4000 10000 AC_ACCOUNT Public Accountant 4200 9000 PR_REP Public Relations Representative 4500 10500 PU_CLERK Purchasing Clerk 2500 5500 PU_MAN Purchasing Manager 8000 15000 SA_MAN Sales Manager 10000 20000 SA_REP Sales Representative 6000 12000 SH_CLERK Shipping Clerk 2500 5500 ST_CLERK Stock Clerk 2000 5000 ST_MAN Stock Manager 5500 8500
Click to view the table contain:
Python Code Editor:
Structure of HR database :
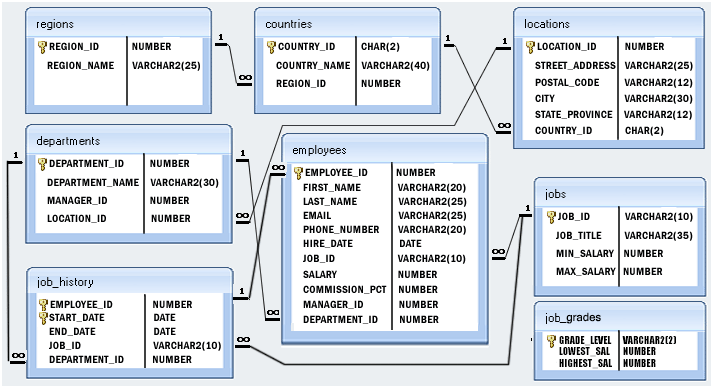
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Pandas program to calculate minimum, maximum and mean salary from employees file.
Next: Write a Pandas program to display the first and last name and date of joining of the employees who is either Sales Representative or Sales Man.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics