Pandas SQL Query: Count the NaN values of all the columns of locations file
14. Count NaN Values of All Columns in LOCATIONS File
Write a Pandas program to count the NaN values of all the columns of locations file.
LOCATIONS.csv
Sample Solution :
Python Code :
import pandas as pd
pd.set_option('display.max_rows', 500)
pd.set_option('display.max_columns', 500)
employees = pd.read_csv(r"EMPLOYEES.csv")
departments = pd.read_csv(r"DEPARTMENTS.csv")
job_history = pd.read_csv(r"JOB_HISTORY.csv")
jobs = pd.read_csv(r"JOBS.csv")
countries = pd.read_csv(r"COUNTRIES.csv")
regions = pd.read_csv(r"REGIONS.csv")
locations = pd.read_csv(r"LOCATIONS.csv")
print("\nNaN values of all the columns of locations file:" )
print(locations.isna().sum())
Sample Output:
NaN values of all the columns of locations file: location_id 0 street_address 0 postal_code 1 city 0 state_province 6 country_id 0 dtype: int64
Click to view the table contain:
For more Practice: Solve these Related Problems:
- Write a Pandas program to count the NaN values in each column of LOCATIONS.csv using isna().sum().
- Write a Pandas program to calculate and display the percentage of NaN values per column in LOCATIONS.csv.
- Write a Pandas program to count NaN values for each column and visualize the counts using a bar chart.
- Write a Pandas program to generate a report that lists each column along with its total NaN count from LOCATIONS.csv.
Go to:
Previous: Write a Pandas program to create a boolean series selecting rows with one or more nulls from locations file.
Next: Write a Pandas program to display the first name, last name, salary and department number for those employees whose first name ends with the letter 'm'.
Python Code Editor:
Structure of HR database :
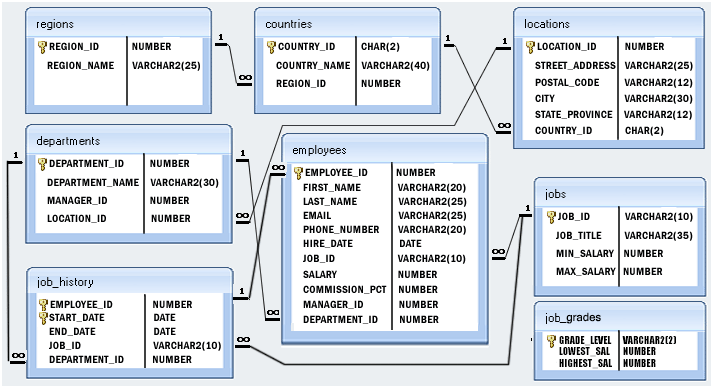
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?