Creating a Pie Chart with Pandas and Matplotlib
Pandas: Visualization Integration Exercise-8 with Solution
Write a Pandas program to create a pie chart using Pandas and Matplotlib.
This exercise shows how to create a pie chart using Pandas and Matplotlib to visualize the proportions of categorical data.
Sample Solution :
Code :
import pandas as pd
import matplotlib.pyplot as plt
# Create a sample DataFrame
df = pd.DataFrame({
'Category': ['A', 'B', 'C', 'D'],
'Values': [30, 25, 20, 25]
})
# Create a pie chart for 'Values' by 'Category'
plt.pie(df['Values'], labels=df['Category'], autopct='%1.1f%%')
# Add a title
plt.title('Category Proportions')
# Display the plot
plt.show()
Output:
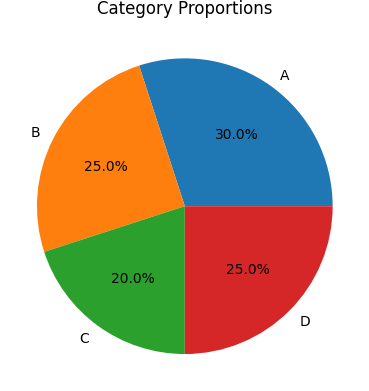
Explanation:
- Created a DataFrame with categories and their values.
- Used plt.pie() to create a pie chart that shows the proportions of each category.
- Added labels and percentage annotations (autopct='%1.1f%%') to the chart.
- Displayed the pie chart with a title.
Python-Pandas Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/pandas/create-a-pie-chart-using-pandas-and-matplotlib.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics