Create a Basic Line Plot with Pandas and Matplotlib
Pandas: Visualization Integration Exercise-1 with Solution
Write a Pandas program to draw a basic line plot with Pandas and Matplotlib.
This exercise demonstrates how to create a simple line plot using Pandas and Matplotlib.
Sample Solution :
Code :
import pandas as pd
import matplotlib.pyplot as plt
# Create a sample DataFrame
df = pd.DataFrame({
'Year': [2017, 2018, 2019, 2020, 2021],
'Sales': [200, 250, 300, 350, 400]
})
# Plot the 'Year' against 'Sales' using a line plot
plt.plot(df['Year'], df['Sales'])
# Add labels and a title
plt.xlabel('Year')
plt.ylabel('Sales')
plt.title('Yearly Sales Trend')
# Display the plot
plt.show()
Output:
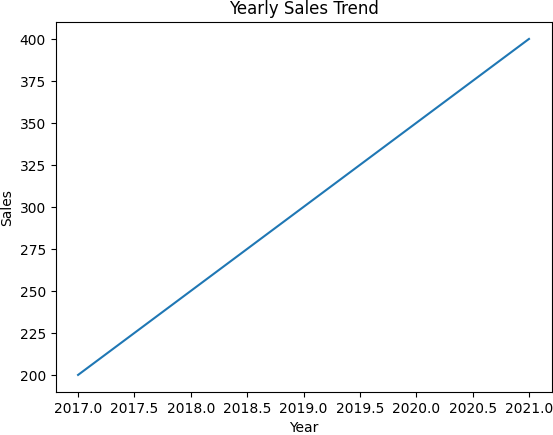
Explanation:
- Created a sample DataFrame with 'Year' and 'Sales' columns.
- Used plt.plot() to plot 'Year' vs 'Sales'.
- Added axis labels and a title to the plot using plt.xlabel(), plt.ylabel(), and plt.title().
- Displayed the plot using plt.show().
Python-Pandas Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics