NumPy: Check whether the numpy array is empty or not
NumPy: Array Object Exercise-95 with Solution
Write a NumPy program to check whether the NumPy array is empty or not.
Pictorial Presentation:
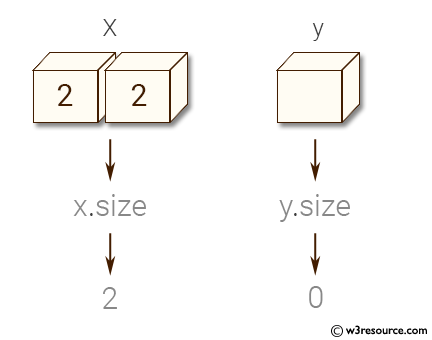
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a NumPy array 'x' containing integers [2, 3]
x = np.array([2, 3])
# Creating an empty NumPy array 'y'
y = np.array([])
# Printing the size of array 'x'
# As 'x' contains 2 elements, its size is 2
print(x.size)
# Printing the size of array 'y'
# 'y' is an empty array, so its size is 0
print(y.size)
Sample Output:
2 0
Explanation:
In the above code –
x = np.array([2, 3]): This line creates a NumPy array 'x' containing the integer values 2 and 3.
y = np.array([]): This line creates an empty NumPy array 'y'.
print(x.size): Print the size of array 'x' using the size attribute. In this case, the size is 2 since 'x' contains two elements.
print(y.size): Print the size of array 'y' using the size attribute. In this case, the size is 0 since 'y' is an empty array.
Python-Numpy Code Editor:
Previous: Write a NumPy program to count the frequency of unique values in numpy array.
Next: Write a NumPy program to divide each row by a vector element.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics