NumPy: Convert a NumPy array into Python list structure
NumPy: Array Object Exercise-80 with Solution
Write a NumPy program to convert a NumPy array into a Python list structure.
Pictorial Presentation:
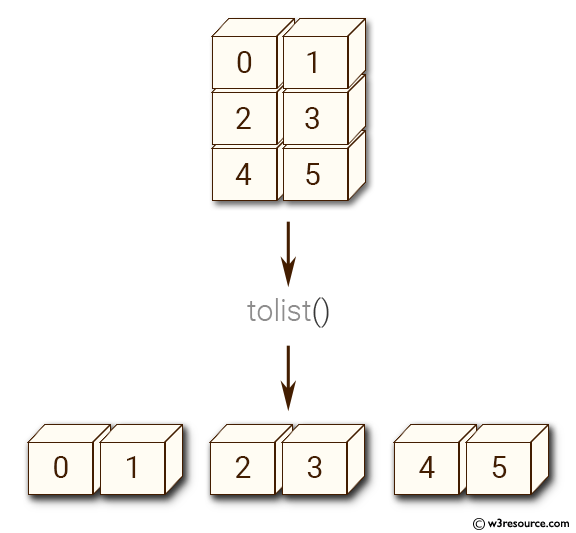
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Creating a 1-dimensional array 'x' with values from 0 to 5 and reshaping it into a 3x2 array
x = np.arange(6).reshape(3, 2)
# Printing a message indicating the original array elements will be shown
print("Original array elements:")
# Printing the original array 'x' with its elements
print(x)
# Printing a message indicating that the array will be converted to a Python list
print("Array to list:")
# Converting the NumPy array 'x' into a Python list using the tolist() method
print(x.tolist())
Sample Output:
Original array elements: [[0 1] [2 3] [4 5]] Array to list: [[0, 1], [2, 3], [4, 5]]
Explanation:
In the above code –
‘x = np.arange(6).reshape(3, 2)’ creates a NumPy array x using np.arange(6), which generates an array with values from 0 to 5. Then, reshape the array into a 3x2 matrix using the reshape method.
print(x.tolist()): Convert the NumPy array ‘x’ to a nested list using the tolist method and print the resulting list.
Python-Numpy Code Editor:
Previous: Write a NumPy program to generate a generic 2D Gaussian-like array.
Next: Write a NumPy program to access an array by column.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics