NumPy: Create a function cube which cubes all the elements of an array
NumPy: Array Object Exercise-76 with Solution
Write a NumPy program to create a function cube for all array elements.
Pictorial Presentation:
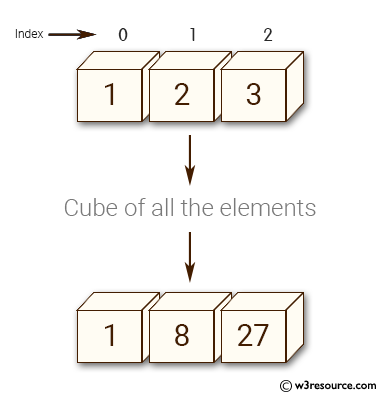
Sample Solution:
Python Code:
# Importing the NumPy library and aliasing it as 'np'
import numpy as np
# Defining a function 'cube' that takes an input 'e'
def cube(e):
# Creating an nditer object 'it' to iterate over 'e' along with an uninitialized 'None' array
it = np.nditer([e, None])
# Iterating through each element 'a' in 'e' and corresponding uninitialized element 'b'
for a, b in it:
# Setting 'b' to the cube of 'a' in each iteration
b[...] = a * a * a
# Returning the resulting array after cube operations from the operands of the iterator
return it.operands[1]
# Calling the 'cube' function with a list [1, 2, 3] as an argument and printing the result
print(cube([1, 2, 3]))
Sample Output:
[ 1 8 27]
Explanation:
In the above code -
- def cube(e):: Define a function called cube that takes a single argument e.
- it = np.nditer([e, None]): Create a NumPy iterator called it that iterates over the input array e and an uninitialized output array of the same shape as e. This is done by passing None as the second element in the list.
- for a, b in it:: Iterate over the elements of e and the uninitialized output array using the iterator it. a represents an element from e, and b represents the corresponding element in the output array.
- b[...] = a*a*a: Cube the value of a and assign it to the corresponding element b in the output array.
- return it.operands[1]: After the loop, return the output array, which is stored as the second operand in the iterator it.
- Finally ‘print(cube([1,2,3]))’ prints the result.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create an array of zeros and three column types (integer, float, character).
Next: Write a NumPy program to create an array of (3, 4) shape and convert the array elements in smaller chunks.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics