NumPy: Interchange two axes of an array
Change Two Array Axes
Write a NumPy program to change two array axes.
Pictorial Presentation:
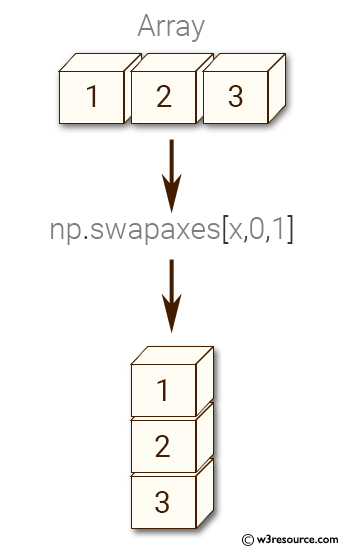
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 2D NumPy array with one row and three columns
x = np.array([[1, 2, 3]])
# Printing the original array
print(x)
# Swapping the axes (dimensions) of the array using 'swapaxes' method
# Swapping axis 0 (rows) with axis 1 (columns)
y = np.swapaxes(x, 0, 1)
# Printing the array after swapping axes
print(y)
Sample Output:
[[1 2 3]] [[1] [2] [3]]
Explanation:
In the above code - x = np.array([[1,2,3]]): This line creates a 2D array x with the given list of lists. The array has a shape of (1, 3).
y = np.swapaxes(x,0,1): The np.swapaxes() function takes three arguments: the input array, and two axis numbers to be swapped. In this case, it swaps axis 0 (rows) with axis 1 (columns) of the array x. As a result, the shape of the new array y is (3, 1).
For more Practice: Solve these Related Problems:
- Write a NumPy program to swap the axes of a 2D array using np.swapaxes and verify the new shape.
- Change the orientation of a row vector into a column vector by interchanging its axes without using np.transpose.
- Utilize np.moveaxis to interchange two specified axes in a multi-dimensional array and compare the resulting shape.
- Create a function that takes an array and two axis indices, then returns the array with those axes swapped.
Go to:
PREV : Find 4th Element of Array
NEXT : Move Array Axes to Alternate Positions
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.