NumPy: Create a 3-D array with ones on the diagonal and zeros elsewhere
3D Array with Diagonal Ones
Write a NumPy program to create a 3-D array with ones on a diagonal and zeros elsewhere.
Pictorial Presentation:
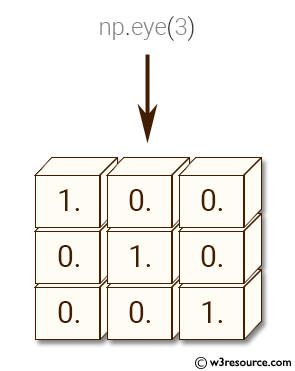
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 3x3 identity matrix using the eye method in NumPy
x = np.eye(3)
# Printing the created identity matrix
print(x)
Sample Output:
[[ 1. 0. 0.] [ 0. 1. 0.] [ 0. 0. 1.]]
Explanation:
The above code demonstrates how to create an identity matrix (a square matrix with ones on the diagonal and zeros elsewhere) using the np.eye() function in NumPy.
x = np.eye(3): np.eye(3This line creates a 3x3 identity matrix x using the np.eye() function. The function takes one argument, which is the number of rows (and columns, since it's a square matrix) in the resulting identity matrix.
print(x): This line prints the identity matrix x:
For more Practice: Solve these Related Problems:
- Create a 3D array where each 2D sub-array is an identity matrix using np.eye in a loop or comprehension.
- Write a function that generates a 3D array with diagonal ones for any given square dimension and depth.
- Validate that every 2D slice of the 3D array contains ones on the main diagonal and zeros elsewhere.
- Extend the concept to create a 3D array where only a specific cross-section diagonal is set to one.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create an array of 10's with the same shape and type of an given array.Next: Write a NumPy program to create a 2-D array whose diagonal equals [4, 5, 6, 8] and 0's elsewhere.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.