NumPy: Create an array of 10's with the same shape and type of a given array
Array of 10s with Given Shape
Write a NumPy program to create an array of 10's with the same shape and type as the given array.
Sample array: x = np.arange(4, dtype=np.int64).
Pictorial Presentation:
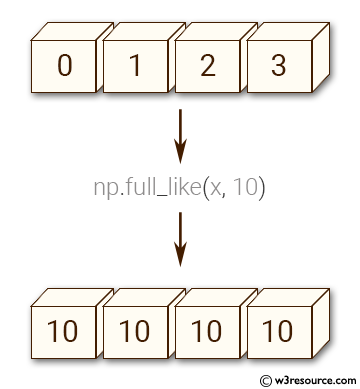
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array with values ranging from 0 to 3 using arange method and specifying the data type as int64
x = np.arange(4, dtype=np.int64)
# Creating a new array 'y' with the same shape and type as array 'x' but filled with the value 10 using full_like method
y = np.full_like(x, 10)
# Printing the new array 'y'
print(y)
Sample Output:
[10 10 10 10]
Explanation:
x = np.arange(4, dtype=np.int64): This line creates a NumPy array ‘x’ containing elements from 0 to 3 (4-1) with a data type of np.int64 (64-bit integer).
y = np.full_like(x, 10): This line creates a new NumPy array ‘y’ with the same shape and data type as ‘x’, but filled with the value 10.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics