NumPy: Create a new shape to an array without changing its data
NumPy: Array Object Exercise-38 with Solution
Write a NumPy program to create another shape from an array without changing its data.
Pictorial Presentation:
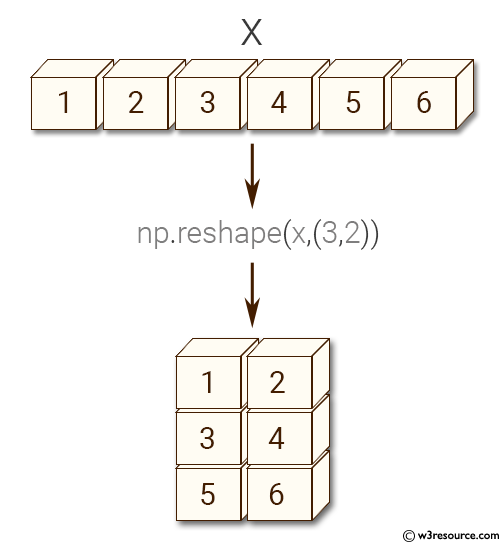
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 1D NumPy array with six elements
x = np.array([1, 2, 3, 4, 5, 6])
# Reshaping the array 'x' into a 3x2 matrix and assigning it to variable 'y'
y = np.reshape(x, (3, 2))
print("Reshape 3x2:") # Printing the description for the reshaped 3x2 array
print(y) # Displaying the reshaped 3x2 array
# Reshaping the array 'x' into a 2x3 matrix and assigning it to variable 'z'
z = np.reshape(x, (2, 3))
print("Reshape 2x3:") # Printing the description for the reshaped 2x3 array
print(z) # Displaying the reshaped 2x3 array
Sample Output:
Reshape 3x2: [[1 2] [3 4] [5 6]] Reshape 2x3: [[1 2 3] [4 5 6]]
Explanation:
In the above code -
x = np.array([1, 2, 3, 4, 5, 6]): This line creates a one-dimensional NumPy array ‘x’ with six elements.
y = np.reshape(x,(3,2)): This line reshapes the ‘x’ array into a new two-dimensional array ‘y’ with 3 rows and 2 columns. The elements from ‘x’ are placed row-wise into the new shape.
print(y): This line prints the reshaped ‘y’ array.
z = np.reshape(x,(2,3)): This line reshapes the ‘x’ array into another two-dimensional array ‘z’ with 2 rows and 3 columns. Again, the elements from ‘x’ are placed row-wise into the new shape.
print(z): This line prints the reshaped ‘z’ array.
Python-Numpy Code Editor:
Previous: Write a NumPy program to create a 2-dimensional array of size 2 x 3 (composed of 4-byte integer elements), also print the shape, type and data type of the array.
Next: Write a NumPy program to change the data type of an array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/numpy/python-numpy-exercise-38.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics