NumPy: Create a contiguous flattened array
Flatten Array
Write a NumPy program to create a contiguous flattened array.
Pictorial Presentation:
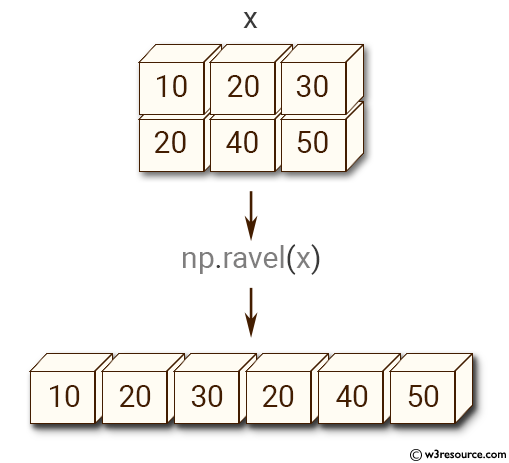
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a 2D NumPy array with two rows and three columns
x = np.array([[10, 20, 30], [20, 40, 50]])
# Displaying the original array
print("Original array:")
print(x)
# Flattening the array 'x' into a 1D array using np.ravel
y = np.ravel(x)
# Displaying the flattened array 'y'
print("New flattened array:")
print(y)
Sample Output:
Original array: [[10 20 30] [20 40 50]] New flattened array: [10 20 30 20 40 50]
Explanation:
In the above exercise -
x = np.array([[10, 20, 30], [20, 40, 50]]): This line creates a two-dimensional NumPy array ‘x’ with two rows and three columns.
print(x): This line prints the ‘x’ array, which has the shape (2, 3) and contains the specified elements.
y = np.ravel(x): This line flattens the two-dimensional array ‘x’ into a one-dimensional array y using the np.ravel() function.
print(y): This line prints the flattened one-dimensional array ‘y’, which contains the elements [10, 20, 30, 20, 40, 50].
Python-Numpy Code Editor:
Previous: Write a NumPy program to change the dimension of an array.Next: Write a NumPy program to create a 2-dimensional array of size 2 x 3 (composed of 4-byte integer elements), also print the shape, type and data type of the array.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics