NumPy: Compare two given arrays
Compare Two Arrays Element-Wise
Write a NumPy program to compare two arrays using NumPy.
Pictorial Presentation:
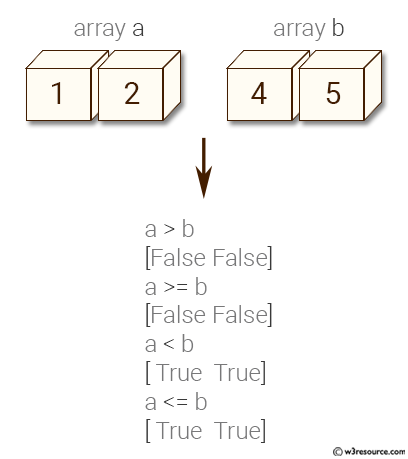
Sample Solution:
Python Code:
Sample Output:
Array a: [1 2] Array b: [4 5] a > b [False False] a >= b [False False] a < b [ True True] a <= b [ True True]
Explanation:
In the above code -
a = np.array([1, 2]): A 1D NumPy array a is created with elements [1, 2].
b = np.array([4, 5]): A 1D NumPy array b is created with elements [4, 5].
print(np.greater(a, b)): The np.greater function compares the elements of a and b element-wise and returns a boolean array with the result of the comparison a > b. In this case, the output is [False False].
print(np.greater_equal(a, b)): The np.greater_equal function compares the elements of a and b element-wise and returns a boolean array with the result of the comparison a >= b. In this case, the output is [False False].
print(np.less(a, b)): The np.less function compares the elements of a and b element-wise and returns a boolean array with the result of the comparison a < b. In this case, the output is [True True].
print(np.less_equal(a, b)): The np.less_equal function compares the elements of a and b element-wise and returns a boolean array with the result of the comparison a <= b. In this case, the output is [True True].
For more Practice: Solve these Related Problems:
- Compare two arrays element-wise using np.equal and verify the resulting boolean array.
- Utilize broadcasting rules to compare arrays of different shapes and check the consistency of the output.
- Create a function that implements element-wise comparison with a tolerance for floating-point numbers using np.isclose.
- Compare the outputs of np.equal and a custom loop-based comparison on identical arrays.
Go to:
PREV : Indices of Max/Min Along Axis
NEXT : Sort Array Along Axes
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.