NumPy: Find the indices of the maximum and minimum values along the given axis of an array
Indices of Max/Min Along Axis
Write a NumPy program to find the indices of the maximum and minimum values along the given axis of an array.
Pictorial Presentation:
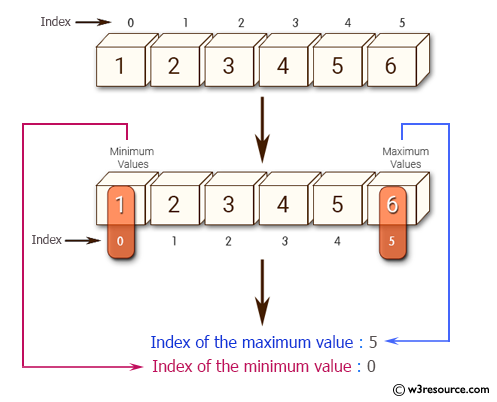
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating a NumPy array
x = np.array([1, 2, 3, 4, 5, 6])
# Displaying the original array
print("Original array: ", x)
# Finding the index of the maximum value in the array
print("Maximum Values: ", np.argmax(x))
# Finding the index of the minimum value in the array
print("Minimum Values: ", np.argmin(x))
Sample Output:
Original array: [1 2 3 4 5 6] Maximum Values: 5 Minimum Values: 0
Explanation:
In the above code -
x = np.array([1, 2, 3, 4, 5, 6]): A 1D NumPy array x is created with elements [1, 2, 3, 4, 5, 6].
print("Maximum Values: ", np.argmax(x)): The np.argmax function finds the index of the maximum value in the array x. In this case, the maximum value is 6, and its index is 5.
The output is: Maximum Values: 5.
print("Minimum Values: ", np.argmin(x)): The np.argmin function finds the index of the minimum value in the array x. In this case, the minimum value is 1, and its index is 0. The output is: Minimum Values: 0.
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics