NumPy: Test whether any array element along a given axis evaluates to True
NumPy: Array Object Exercise-24 with Solution
Write a NumPy program to test whether any array element along a given axis evaluates to True.
Note: 0 evaluates to False in python.
Pictorial Presentation:
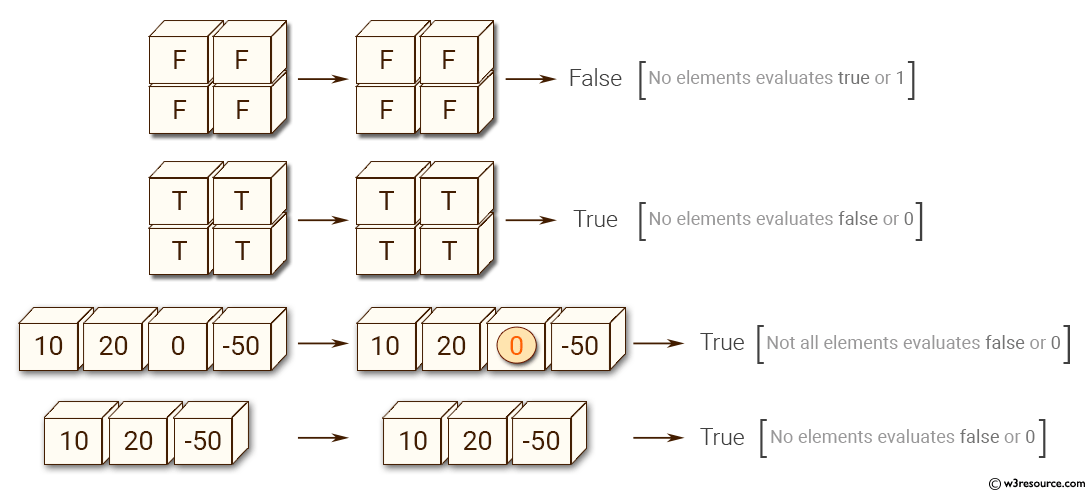
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Checking if any elements along a specified axis evaluate to True or non-zero
print(np.any([[False,False],[False,False]])) # Evaluates to False because all elements in the array are False
print(np.any([[True,True],[True,True]])) # Evaluates to True because at least one element in the array is True
# Checking if any elements in an iterable evaluate to True or non-zero
print(np.any([10, 20, 0, -50])) # Evaluates to True because at least one element in the list is non-zero and evaluates to True
print(np.any([10, 20, -50])) # Evaluates to True because all elements in the list are non-zero and evaluate to True
Sample Output:
False True True True
Explanation:
In the above code –
print(np.any([[False,False],[False,False]])): np.any checks if any element of the input array is True. In this case, all elements are False, so the output is False.
print(np.any([[True,True],[True,True]])): np.any checks if any element of the input array is True. In this case, all elements are True, so the output is True.
print(np.any([10, 20, 0, -50])): np.any checks if any element of the input array is non-zero. In this case, there are non-zero elements in the array (10, 20, and -50), so the output is True.
print(np.any([10, 20, -50])): np.any checks if any element of the input array is non-zero. In this case, all elements are non-zero, so the output is True.
Python-Numpy Code Editor:
Previous: Write a NumPy program to test if all elements in an array evaluate to True.
Next: Write a NumPy program to construct an array by repeating.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics