NumPy: Find common values between two arrays
Common Values in Two Arrays
Write a NumPy program to find common values between two arrays.
Pictorial Presentation:
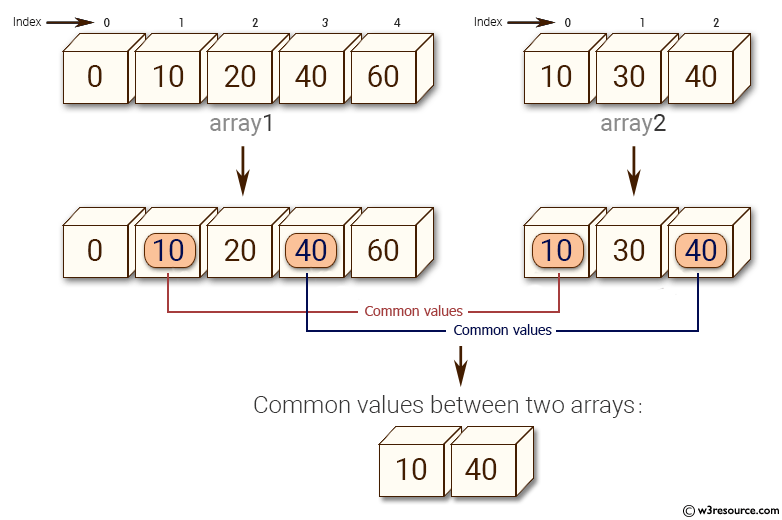
Sample Solution:-
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating an array 'array1' using NumPy
array1 = np.array([0, 10, 20, 40, 60])
# Printing the contents of 'array1'
print("Array1: ", array1)
# Creating a Python list 'array2'
array2 = [10, 30, 40]
# Printing the contents of 'array2'
print("Array2: ", array2)
# Finding the common values between 'array1' and 'array2'
print("Common values between two arrays:")
print(np.intersect1d(array1, array2))
Sample Output:
Array1: [ 0 10 20 40 60] Array2: [10, 30, 40] Common values between two arrays: [10 40]
Explanation:
In the above code –
array1 = np.array([0, 10, 20, 40, 60]): Creates a NumPy array with elements 0, 10, 20, 40, and 60.
array2 = [10, 30, 40]: Creates a Python list with elements 10, 30, and 40.
print(np.intersect1d(array1, array2)): The np.intersect1d function returns a sorted array containing the common elements of the two input arrays, ‘array1’ and ‘array2’. In this case, the common elements are 10 and 40, so the output will be [10 40].
For more Practice: Solve these Related Problems:
- Find the intersection of two arrays using np.intersect1d and ensure the result is sorted.
- Handle duplicate values by extracting unique common elements between two arrays.
- Create a function to return both the common values and their counts in each array.
- Compare two arrays of different dimensions and extract only the common scalar values.
Go to:
PREV : 1D Array Element Check in Another Array
NEXT : Unique Elements of Array
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.