NumPy: Test whether each element of a 1-D array
1D Array Element Check in Another Array
Write a NumPy program to test whether each element of a 1-D array is also present in a second array.
Pictorial Presentation:
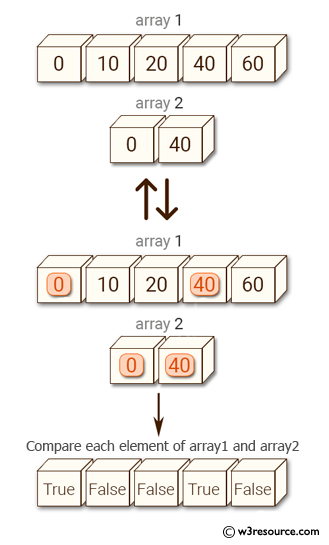
Sample Solution:
Python Code:
# Importing the NumPy library with an alias 'np'
import numpy as np
# Creating an array 'array1' using NumPy
array1 = np.array([0, 10, 20, 40, 60])
# Printing the contents of 'array1'
print("Array1: ", array1)
# Creating a Python list 'array2'
array2 = [0, 40]
# Printing the contents of 'array2'
print("Array2: ", array2)
# Comparing each element of 'array1' with 'array2'
print("Compare each element of array1 and array2")
print(np.in1d(array1, array2))
Sample Output:
Array1: [ 0 10 20 40 60] Array2: [0, 40] Compare each element of array1 and array2 [ True False False True False]
Explanation:
In the above code –
array1 = np.array([0, 10, 20, 40, 60]): Creates a NumPy array with elements 0, 10, 20, 40, and 60.
array2 = [0, 40]: Creates a Python list with elements 0 and 40.
print(np.in1d(array1, array2)): The np.in1d function checks if each element of ‘array1’ is present in ‘array2’. It returns a boolean array of the same shape as ‘array1’, with True at positions where the element is present in ‘array2’ and False otherwise. The output will be [ True False False True False]
Python-Numpy Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics