Python: Shuffle the elements of a given list
6. List Shuffle
Write a Python program to shuffle the elements of a given list.
Use random.shuffle()
Sample Solution:
Python Code:
import random
nums = [1, 2, 3, 4, 5]
print("Original list:")
print(nums)
random.shuffle(nums)
print("Shuffle list:")
print(nums)
words = ['red', 'black', 'green', 'blue']
print("\nOriginal list:")
print(words)
random.shuffle(words)
print("Shuffle list:")
print(words)
Sample Output:
Original list: [1, 2, 3, 4, 5] Shuffle list: [4, 2, 1, 5, 3] Original list: ['red', 'black', 'green', 'blue'] Shuffle list: ['green', 'blue', 'black', 'red']
Flowchart:
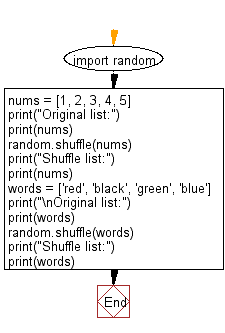
For more Practice: Solve these Related Problems:
- Write a Python program to shuffle a given list of numbers using random.shuffle() and then verify that the original list order has changed.
- Write a Python script to shuffle a list of strings and then print the list before and after shuffling.
- Write a Python function to randomize the order of elements in a list of tuples and return the shuffled list.
- Write a Python program to shuffle a list of 20 random integers and then compute the difference between the first element of the original and shuffled lists.
Go to:
Previous: Write a Python program to generate a random integer between 0 and 6 - excluding 6, random integer between 5 and 10 - excluding 10, random integer between 0 and 10, with a step of 3 and random date between two dates.
Next: Write a Python program to generate a float between 0 and 1, inclusive and generate a random float within a specific range.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.