Python: Configure the rounding to round up and round down a given decimal value
2. Rounding Up/Down Configuration
Write a Python program to configure rounding to round up and round down a given decimal value. Use decimal.Decimal
Sample Solution:
Python Code:
import decimal
print("Configure the rounding to round up:")
decimal.getcontext().prec = 1
decimal.getcontext().rounding = decimal.ROUND_UP
print(decimal.Decimal(30) / decimal.Decimal(4))
print("\nConfigure the rounding to round down:")
decimal.getcontext().prec = 3
decimal.getcontext().rounding = decimal.ROUND_DOWN
print(decimal.Decimal(30) / decimal.Decimal(4))
print("\nConfigure the rounding to round up:")
print(decimal.Decimal('8.325').quantize(decimal.Decimal('.01'), rounding=decimal.ROUND_UP))
print("\nConfigure the rounding to round down:")
print(decimal.Decimal('8.325').quantize(decimal.Decimal('.01'), rounding=decimal.ROUND_DOWN))
Sample Output:
Configure the rounding to round up: 8 Configure the rounding to round down: 7.5 Configure the rounding to round up: 8.33 Configure the rounding to round down: 8.32
Flowchart:
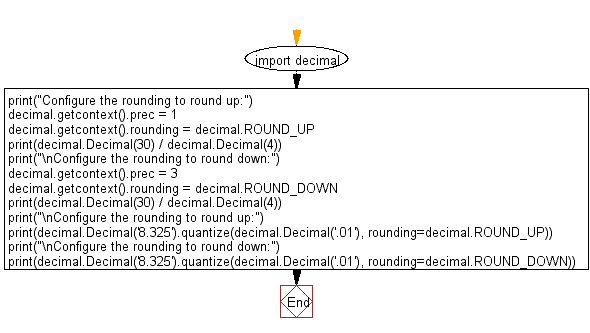
For more Practice: Solve these Related Problems:
- Write a Python program that sets the rounding mode to ROUND_UP and ROUND_DOWN for a given Decimal, then prints the results of rounding a sample value in each mode.
- Write a Python function to configure Decimal rounding modes to round up and down, apply them to a list of Decimal values, and print the outcomes.
- Write a Python script that creates a Decimal value and demonstrates the difference between rounding up and rounding down using the local context.
- Write a Python program to compare the effects of ROUND_UP and ROUND_DOWN on several Decimal values and output the differences in a formatted table.
Go to:
Previous: Write a Python program to construct a Decimal from a float and a Decimal from a string. Also represent the Decimal value as a tuple.
Next: Write a Python program to round a Decimal value to the nearest multiple of 0.10, unless already an exact multiple of 0.05.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.