Python: Write (without writing separate lines between rows) and read a CSV file with specified delimiter
6. CSV Writer with Custom Delimiter
Write a Python program to write (without writing separate lines between rows) and read a CSV file with a specified delimiter. Use csv.reader
Sample Solution:
Python Code:
import csv
fw = open("test.csv", "w", newline='')
writer = csv.writer(fw, delimiter = ",")
writer.writerow(["a","b","c"])
writer.writerow(["d","e","f"])
writer.writerow(["g","h","i"])
fw.close()
fr = open("test.csv", "r")
csv = csv.reader(fr, delimiter = ",")
for row in csv:
print(row)
fr.close()
Sample Output:
['a', 'b', 'c'] ['d', 'e', 'f'] ['g', 'h', 'i']
Flowchart:
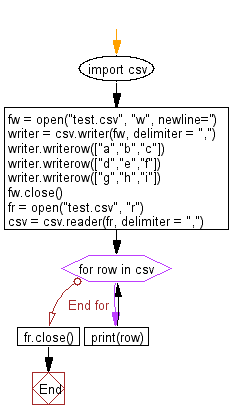
For more Practice: Solve these Related Problems:
- Write a Python program to write a list of lists to a CSV file with a semicolon as the delimiter, then read and print the contents using csv.reader.
- Write a Python script to generate a CSV file using a pipe (|) delimiter without extra newlines, then read it back and display each row.
- Write a Python function that writes data to a CSV file with a custom delimiter, reads the file using csv.reader, and verifies the delimiter by printing the parsed rows.
- Write a Python program to create a CSV file with a colon (:) delimiter, then open the file with csv.reader and print each row to confirm the delimiter is used.
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to skip the headers of a given CSV file.
Next: Write a Python program to write dictionaries and a list of dictionaries to a given CSV file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.