Python: Read the current line from a given CSV file
Write a Python program to read the current line from a given CSV file. Use csv.reader
open(file) function open the "employees.csv" with file as the "f". Call csv.reader(f) to generate an object which will iterate over each line in the given "employees.csv" file. Pass in the reader object to next(csv_reader) to get a single line from the .csv file.
Sample Solution:
Python Code:
Sample Output:
['employ_id', 'first_name', 'last_name', 'email', 'phone_number', 'hire_date', 'job_id', 'salary', 'commission_pct', 'manager_id', 'department_id'] ['100', 'Steven', 'King', 'SKING', '515.123.4567', '1987-06-17', 'AD_PRES', '24000', '', '', '90'] ['101', 'Neena', 'Kochhar', 'NKOCHHAR', '515.123.4568', '1987-06-18', 'AD_VP', '17000', '', '100', '90']
Flowchart:
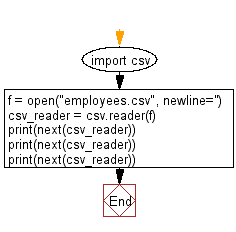
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to parse a given CSV string and get the list of lists of string values.
Next: Write a Python program to skip the headers of a given CSV file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics