Python: Create a deep copy of a given dictionary
Python module: Exercise-4 with Solution
Write a Python program to create a deep copy of a given dictionary. Use copy.copy
Sample Solution:
Python Code:
import copy
nums_x = {"a":1, "b":2, 'cc':{"c":3}}
print("Original dictionary: ", nums_x)
nums_y = copy.deepcopy(nums_x)
print("\nDeep copy of the said list:")
print(nums_y)
print("\nChange the value of an element of the original dictionary:")
nums_x["cc"]["c"] = 10
print(nums_x)
print("\nSecond dictionary (Deep copy):")
print(nums_y)
nums = {"x":1, "y":2, 'zz':{"z":3}}
nums_copy = copy.deepcopy(nums)
print("\nOriginal dictionary :")
print(nums)
print("\nDeep copy of the said list:")
print(nums_copy)
print("\nChange the value of an element of the original dictionary:")
nums["zz"]["z"] = 10
print("\nFirst dictionary:")
print(nums)
print("\nSecond dictionary (Deep copy):")
print(nums_copy)
Sample Output:
Original dictionary: {'a': 1, 'b': 2, 'cc': {'c': 3}} Deep copy of the said list: {'a': 1, 'b': 2, 'cc': {'c': 3}} Change the value of an element of the original dictionary: {'a': 1, 'b': 2, 'cc': {'c': 10}} Second dictionary (Deep copy): {'a': 1, 'b': 2, 'cc': {'c': 3}} Original dictionary : {'x': 1, 'y': 2, 'zz': {'z': 3}} Deep copy of the said list: {'x': 1, 'y': 2, 'zz': {'z': 3}} Change the value of an element of the original dictionary: First dictionary: {'x': 1, 'y': 2, 'zz': {'z': 10}} Second dictionary (Deep copy): {'x': 1, 'y': 2, 'zz': {'z': 3}}
Flowchart:
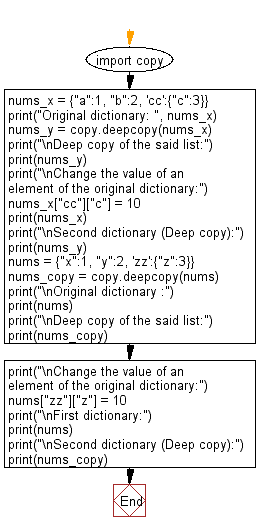
Python Code Editor:
Have another way to solve this solution? Contribute your code (and comments) through Disqus.
Previous: Write a Python program to create a shallow copy of a given dictionary.
Next: Python built-in Modules Exercise Home.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
It will be nice if you may share this link in any developer community or anywhere else, from where other developers may find this content. Thanks.
https://w3resource.com/python-exercises/modules/python-module-copy-exercise-4.php
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics